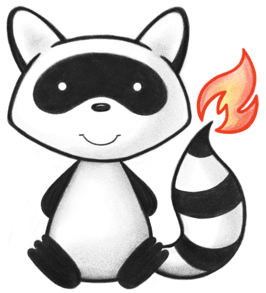
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.interceptor.api.Hook; 023import ca.uhn.fhir.interceptor.api.Pointcut; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026 027/** 028 * Server interceptor with added methods which can be called within the lifecycle of 029 * write operations (create/update/delete) or within transaction and batch 030 * operations that call these sub-operations. 031 * 032 * @see ServerOperationInterceptorAdapter 033 * @deprecated Ths interface is no longer neccessary as of HAPI FHIR 3.8.0 - You can create 034 * interceptor methods that are declared using the {@link Hook} annotation without needing 035 * to implement any interceptor 036 */ 037@Deprecated 038public interface IServerOperationInterceptor extends IServerInterceptor { 039 040 /** 041 * This method is called by the server immediately after a resource has 042 * been created, within the database transaction scope of the operation. 043 * <p> 044 * If an exception is thrown by an interceptor during this method, 045 * the transaction will be rolled back. 046 * </p> 047 */ 048 @Hook(Pointcut.STORAGE_PRECOMMIT_RESOURCE_CREATED) 049 void resourceCreated(RequestDetails theRequest, IBaseResource theResource); 050 051 /** 052 * This method is called by the server immediately after a resource has 053 * been deleted, within the database transaction scope of the operation. 054 * <p> 055 * If an exception is thrown by an interceptor during this method, 056 * the transaction will be rolled back. 057 * </p> 058 */ 059 @Hook(Pointcut.STORAGE_PRECOMMIT_RESOURCE_DELETED) 060 void resourceDeleted(RequestDetails theRequest, IBaseResource theResource); 061 062 /** 063 * This method is called by the server immediately before a resource is about 064 * to be created, within the database transaction scope of the operation. 065 * <p> 066 * This method may be used to modify the resource 067 * </p> 068 * <p> 069 * If an exception is thrown by an interceptor during this method, 070 * the transaction will be rolled back. 071 * </p> 072 * 073 * @param theResource The resource that has been provided by the client as the payload 074 * to create. Interceptors may modify this 075 * resource, and modifications will affect what is saved in the database. 076 */ 077 @Hook(Pointcut.STORAGE_PRESTORAGE_RESOURCE_CREATED) 078 void resourcePreCreate(RequestDetails theRequest, IBaseResource theResource); 079 080 /** 081 * This method is called by the server immediately before a resource is about 082 * to be deleted, within the database transaction scope of the operation. 083 * <p> 084 * If an exception is thrown by an interceptor during this method, 085 * the transaction will be rolled back. 086 * </p> 087 * 088 * @param theResource The resource which is about to be deleted 089 */ 090 @Hook(Pointcut.STORAGE_PRESTORAGE_RESOURCE_DELETED) 091 void resourcePreDelete(RequestDetails theRequest, IBaseResource theResource); 092 093 /** 094 * This method is called by the server immediately before a resource is about 095 * to be updated, within the database transaction scope of the operation. 096 * <p> 097 * This method may be used to modify the resource 098 * </p> 099 * <p> 100 * If an exception is thrown by an interceptor during this method, 101 * the transaction will be rolled back. 102 * </p> 103 * 104 * @param theOldResource The previous version of the resource, or <code>null</code> if this is not available. Interceptors should be able to handle situations where this is null, since it is not always 105 * convenient or possible to provide a value for this field, but servers should try to populate it. 106 * @param theNewResource The resource that has been provided by the client as the payload 107 * to update to the resource to. Interceptors may modify this 108 * resource, and modifications will affect what is saved in the database. 109 */ 110 @Hook(Pointcut.STORAGE_PRESTORAGE_RESOURCE_UPDATED) 111 void resourcePreUpdate(RequestDetails theRequest, IBaseResource theOldResource, IBaseResource theNewResource); 112 113 /** 114 * @deprecated Deprecated in HAPI FHIR 3.0.0 in favour of {@link #resourceUpdated(RequestDetails, IBaseResource, IBaseResource)} 115 */ 116 @Deprecated 117 @Hook(Pointcut.STORAGE_PRECOMMIT_RESOURCE_UPDATED) 118 void resourceUpdated(RequestDetails theRequest, IBaseResource theResource); 119 120 /** 121 * This method is called by the server immediately after a resource has 122 * been created, within the database transaction scope of the operation. 123 * <p> 124 * If an exception is thrown by an interceptor during this method, 125 * the transaction will be rolled back. 126 * </p> 127 * 128 * @param theOldResource The resource as it was before the update, or <code>null</code> if this is not available. Interceptors should be able to handle situations where this is null, since it is not always 129 * convenient or possible to provide a value for this field, but servers should try to populate it. 130 * @param theNewResource The resource as it will be after the update 131 */ 132 @Hook(Pointcut.STORAGE_PRECOMMIT_RESOURCE_UPDATED) 133 void resourceUpdated(RequestDetails theRequest, IBaseResource theOldResource, IBaseResource theNewResource); 134}