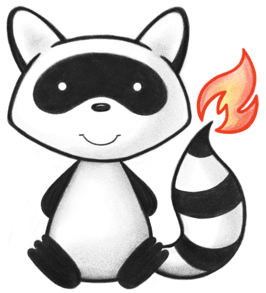
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.model.api.TagList; 023import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.api.server.ResponseDetails; 026import ca.uhn.fhir.rest.server.exceptions.AuthenticationException; 027import ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException; 028import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 029import jakarta.servlet.ServletException; 030import jakarta.servlet.http.HttpServletRequest; 031import jakarta.servlet.http.HttpServletResponse; 032import org.hl7.fhir.instance.model.api.IBaseResource; 033 034import java.io.IOException; 035 036/** 037 * Base class for {@link IServerInterceptor} implementations. Provides a No-op implementation 038 * of all methods, always returning <code>true</code> 039 */ 040public class InterceptorAdapter implements IServerInterceptor { 041 042 @Override 043 public boolean handleException( 044 RequestDetails theRequestDetails, 045 BaseServerResponseException theException, 046 HttpServletRequest theServletRequest, 047 HttpServletResponse theServletResponse) 048 throws ServletException, IOException { 049 return true; 050 } 051 052 @Override 053 public boolean incomingRequestPostProcessed( 054 RequestDetails theRequestDetails, HttpServletRequest theRequest, HttpServletResponse theResponse) 055 throws AuthenticationException { 056 return true; 057 } 058 059 @Override 060 public void incomingRequestPreHandled(RestOperationTypeEnum theOperation, RequestDetails theProcessedRequest) { 061 // nothing 062 } 063 064 @Override 065 public boolean incomingRequestPreProcessed(HttpServletRequest theRequest, HttpServletResponse theResponse) { 066 return true; 067 } 068 069 @Override 070 public boolean outgoingResponse(RequestDetails theRequestDetails) { 071 ServletRequestDetails details = (ServletRequestDetails) theRequestDetails; 072 return outgoingResponse(theRequestDetails, details.getServletRequest(), details.getServletResponse()); 073 } 074 075 @Override 076 public boolean outgoingResponse( 077 RequestDetails theRequestDetails, 078 HttpServletRequest theServletRequest, 079 HttpServletResponse theServletResponse) 080 throws AuthenticationException { 081 return true; 082 } 083 084 @Override 085 public boolean outgoingResponse(RequestDetails theRequestDetails, IBaseResource theResponseObject) { 086 ServletRequestDetails details = (ServletRequestDetails) theRequestDetails; 087 return outgoingResponse(details, theResponseObject, details.getServletRequest(), details.getServletResponse()); 088 } 089 090 @Override 091 public boolean outgoingResponse( 092 RequestDetails theRequestDetails, 093 IBaseResource theResponseObject, 094 HttpServletRequest theServletRequest, 095 HttpServletResponse theServletResponse) 096 throws AuthenticationException { 097 return true; 098 } 099 100 @Override 101 public boolean outgoingResponse( 102 RequestDetails theRequestDetails, 103 ResponseDetails theResponseDetails, 104 HttpServletRequest theServletRequest, 105 HttpServletResponse theServletResponse) 106 throws AuthenticationException { 107 return true; 108 } 109 110 @Override 111 public boolean outgoingResponse(RequestDetails theRequestDetails, TagList theResponseObject) { 112 ServletRequestDetails details = (ServletRequestDetails) theRequestDetails; 113 return outgoingResponse(details, theResponseObject, details.getServletRequest(), details.getServletResponse()); 114 } 115 116 @Override 117 public boolean outgoingResponse( 118 RequestDetails theRequestDetails, 119 TagList theResponseObject, 120 HttpServletRequest theServletRequest, 121 HttpServletResponse theServletResponse) 122 throws AuthenticationException { 123 return true; 124 } 125 126 @Override 127 public BaseServerResponseException preProcessOutgoingException( 128 RequestDetails theRequestDetails, Throwable theException, HttpServletRequest theServletRequest) 129 throws ServletException { 130 return null; 131 } 132 133 @Override 134 public void processingCompletedNormally(ServletRequestDetails theRequestDetails) { 135 // nothing 136 } 137}