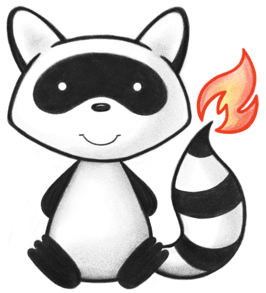
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.interceptor.api.Hook; 023import ca.uhn.fhir.interceptor.api.Pointcut; 024import ca.uhn.fhir.rest.api.EncodingEnum; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import ca.uhn.fhir.rest.server.RestfulServerUtils; 027import ca.uhn.fhir.rest.server.exceptions.AuthenticationException; 028import ca.uhn.fhir.rest.server.exceptions.UnprocessableEntityException; 029import ca.uhn.fhir.rest.server.method.ResourceParameter; 030import ca.uhn.fhir.validation.FhirValidator; 031import ca.uhn.fhir.validation.ResultSeverityEnum; 032import ca.uhn.fhir.validation.ValidationResult; 033import jakarta.servlet.http.HttpServletRequest; 034import jakarta.servlet.http.HttpServletResponse; 035 036import java.nio.charset.Charset; 037 038import static org.apache.commons.lang3.StringUtils.isBlank; 039 040/** 041 * This interceptor intercepts each incoming request and if it contains a FHIR resource, validates that resource. The 042 * interceptor may be configured to run any validator modules, and will then add headers to the response or fail the 043 * request with an {@link UnprocessableEntityException HTTP 422 Unprocessable Entity}. 044 */ 045public class RequestValidatingInterceptor extends BaseValidatingInterceptor<String> { 046 047 /** 048 * X-HAPI-Request-Validation 049 */ 050 public static final String DEFAULT_RESPONSE_HEADER_NAME = "X-FHIR-Request-Validation"; 051 052 private static final org.slf4j.Logger ourLog = 053 org.slf4j.LoggerFactory.getLogger(RequestValidatingInterceptor.class); 054 private boolean myAddValidationResultsToResponseOperationOutcome = true; 055 056 @Override 057 ValidationResult doValidate(FhirValidator theValidator, String theRequest) { 058 return theValidator.validateWithResult(theRequest); 059 } 060 061 @Hook(Pointcut.SERVER_INCOMING_REQUEST_POST_PROCESSED) 062 public boolean incomingRequestPostProcessed( 063 RequestDetails theRequestDetails, HttpServletRequest theRequest, HttpServletResponse theResponse) 064 throws AuthenticationException { 065 EncodingEnum encoding = RestfulServerUtils.determineRequestEncodingNoDefault(theRequestDetails); 066 if (encoding == null) { 067 ourLog.trace("Incoming request does not appear to be FHIR, not going to validate"); 068 return true; 069 } 070 071 Charset charset = ResourceParameter.determineRequestCharset(theRequestDetails); 072 String requestText = new String(theRequestDetails.loadRequestContents(), charset); 073 074 if (isBlank(requestText)) { 075 ourLog.trace("Incoming request does not have a body"); 076 return true; 077 } 078 079 ValidationResult validationResult = validate(requestText, theRequestDetails); 080 081 if (myAddValidationResultsToResponseOperationOutcome) { 082 addValidationResultToRequestDetails(theRequestDetails, validationResult); 083 } 084 085 return true; 086 } 087 088 /** 089 * If set to {@literal true} (default is true), the validation results 090 * will be added to the OperationOutcome being returned to the client, 091 * unless the response being returned is not an OperationOutcome 092 * to begin with (e.g. if the client has requested 093 * <code>Return: prefer=representation</code>) 094 */ 095 public boolean isAddValidationResultsToResponseOperationOutcome() { 096 return myAddValidationResultsToResponseOperationOutcome; 097 } 098 099 /** 100 * If set to {@literal true} (default is true), the validation results 101 * will be added to the OperationOutcome being returned to the client, 102 * unless the response being returned is not an OperationOutcome 103 * to begin with (e.g. if the client has requested 104 * <code>Return: prefer=representation</code>) 105 */ 106 public void setAddValidationResultsToResponseOperationOutcome( 107 boolean theAddValidationResultsToResponseOperationOutcome) { 108 myAddValidationResultsToResponseOperationOutcome = theAddValidationResultsToResponseOperationOutcome; 109 } 110 111 @Override 112 String provideDefaultResponseHeaderName() { 113 return DEFAULT_RESPONSE_HEADER_NAME; 114 } 115 116 /** 117 * Sets the name of the response header to add validation failures to 118 * 119 * @see #DEFAULT_RESPONSE_HEADER_NAME 120 * @see #setAddResponseHeaderOnSeverity(ResultSeverityEnum) 121 */ 122 @Override 123 public void setResponseHeaderName(String theResponseHeaderName) { 124 super.setResponseHeaderName(theResponseHeaderName); 125 } 126}