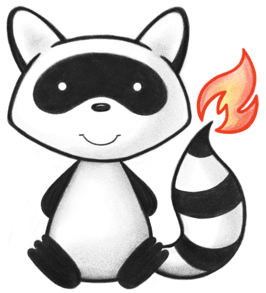
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.context.BaseRuntimeChildDefinition; 023import ca.uhn.fhir.context.BaseRuntimeElementCompositeDefinition; 024import ca.uhn.fhir.context.BaseRuntimeElementDefinition; 025import ca.uhn.fhir.context.RuntimePrimitiveDatatypeDefinition; 026import ca.uhn.fhir.context.support.IValidationSupport; 027import ca.uhn.fhir.context.support.LookupCodeRequest; 028import ca.uhn.fhir.context.support.ValidationSupportContext; 029import ca.uhn.fhir.interceptor.api.Hook; 030import ca.uhn.fhir.interceptor.api.Pointcut; 031import ca.uhn.fhir.rest.api.server.RequestDetails; 032import ca.uhn.fhir.util.FhirTerser; 033import ca.uhn.fhir.util.IModelVisitor; 034import org.hl7.fhir.instance.model.api.IBase; 035import org.hl7.fhir.instance.model.api.IBaseResource; 036import org.hl7.fhir.instance.model.api.IPrimitiveType; 037 038import java.util.List; 039import java.util.Objects; 040 041import static ca.uhn.fhir.rest.server.interceptor.InterceptorOrders.RESPONSE_TERMINOLOGY_DISPLAY_POPULATION_INTERCEPTOR; 042import static org.apache.commons.lang3.StringUtils.isBlank; 043import static org.apache.commons.lang3.StringUtils.isNotBlank; 044 045/** 046 * This interceptor looks for coded data ( 047 * 048 * @since 5.4.0 049 */ 050public class ResponseTerminologyDisplayPopulationInterceptor extends BaseResponseTerminologyInterceptor { 051 052 private final BaseRuntimeChildDefinition myCodingSystemChild; 053 private final BaseRuntimeChildDefinition myCodingCodeChild; 054 private final Class<? extends IBase> myCodingType; 055 private final BaseRuntimeElementCompositeDefinition<?> myCodingDefinitition; 056 private final BaseRuntimeChildDefinition myCodingDisplayChild; 057 private final RuntimePrimitiveDatatypeDefinition myStringDefinition; 058 059 /** 060 * Constructor 061 * 062 * @param theValidationSupport The validation support module 063 */ 064 public ResponseTerminologyDisplayPopulationInterceptor(IValidationSupport theValidationSupport) { 065 super(theValidationSupport); 066 067 myCodingDefinitition = (BaseRuntimeElementCompositeDefinition<?>) 068 Objects.requireNonNull(myContext.getElementDefinition("Coding")); 069 myCodingType = myCodingDefinitition.getImplementingClass(); 070 myCodingSystemChild = myCodingDefinitition.getChildByName("system"); 071 myCodingCodeChild = myCodingDefinitition.getChildByName("code"); 072 myCodingDisplayChild = myCodingDefinitition.getChildByName("display"); 073 074 myStringDefinition = (RuntimePrimitiveDatatypeDefinition) myContext.getElementDefinition("string"); 075 } 076 077 @Hook(value = Pointcut.SERVER_OUTGOING_RESPONSE, order = RESPONSE_TERMINOLOGY_DISPLAY_POPULATION_INTERCEPTOR) 078 public void handleResource(RequestDetails theRequestDetails, IBaseResource theResource) { 079 List<IBaseResource> resources = toListForProcessing(theRequestDetails, theResource); 080 081 FhirTerser terser = myContext.newTerser(); 082 for (IBaseResource nextResource : resources) { 083 terser.visit(nextResource, new MappingVisitor()); 084 } 085 } 086 087 private class MappingVisitor implements IModelVisitor { 088 089 @Override 090 public void acceptElement( 091 IBaseResource theResource, 092 IBase theElement, 093 List<String> thePathToElement, 094 BaseRuntimeChildDefinition theChildDefinition, 095 BaseRuntimeElementDefinition<?> theDefinition) { 096 if (myCodingType.isAssignableFrom(theElement.getClass())) { 097 String system = myCodingSystemChild 098 .getAccessor() 099 .getFirstValueOrNull(theElement) 100 .map(t -> (IPrimitiveType<?>) t) 101 .map(t -> t.getValueAsString()) 102 .orElse(null); 103 String code = myCodingCodeChild 104 .getAccessor() 105 .getFirstValueOrNull(theElement) 106 .map(t -> (IPrimitiveType<?>) t) 107 .map(t -> t.getValueAsString()) 108 .orElse(null); 109 if (isBlank(system) || isBlank(code)) { 110 return; 111 } 112 113 String display = myCodingDisplayChild 114 .getAccessor() 115 .getFirstValueOrNull(theElement) 116 .map(t -> (IPrimitiveType<?>) t) 117 .map(t -> t.getValueAsString()) 118 .orElse(null); 119 if (isNotBlank(display)) { 120 return; 121 } 122 123 ValidationSupportContext validationSupportContext = new ValidationSupportContext(myValidationSupport); 124 if (myValidationSupport.isCodeSystemSupported(validationSupportContext, system)) { 125 126 IValidationSupport.LookupCodeResult lookupCodeResult = myValidationSupport.lookupCode( 127 validationSupportContext, new LookupCodeRequest(system, code)); 128 if (lookupCodeResult != null && lookupCodeResult.isFound()) { 129 String newDisplay = lookupCodeResult.getCodeDisplay(); 130 IPrimitiveType<?> newString = myStringDefinition.newInstance(newDisplay); 131 myCodingDisplayChild.getMutator().addValue(theElement, newString); 132 } 133 } 134 } 135 } 136 } 137}