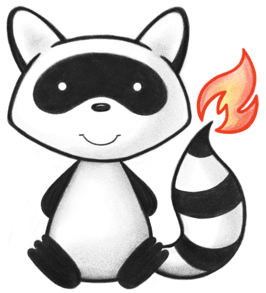
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.context.support.IValidationSupport; 023import ca.uhn.fhir.interceptor.api.Hook; 024import ca.uhn.fhir.interceptor.api.Pointcut; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027 028import java.util.List; 029import java.util.Map; 030 031import static ca.uhn.fhir.rest.server.interceptor.InterceptorOrders.RESPONSE_TERMINOLOGY_TRANSLATION_INTERCEPTOR; 032 033/** 034 * This interceptor leverages ConceptMap resources stored in the repository to automatically map 035 * terminology from one CodeSystem to another at runtime, in resources that are being 036 * returned by the server. 037 * <p> 038 * Mappings are applied only if they are explicitly configured in the interceptor via 039 * the {@link #addMappingSpecification(String, String)} method. 040 * </p> 041 * 042 * @since 5.4.0 043 */ 044public class ResponseTerminologyTranslationInterceptor extends BaseResponseTerminologyInterceptor { 045 046 private final ResponseTerminologyTranslationSvc myResponseTerminologyTranslationSvc; 047 048 /** 049 * Constructor 050 * 051 * @param theValidationSupport The validation support module 052 */ 053 public ResponseTerminologyTranslationInterceptor( 054 IValidationSupport theValidationSupport, 055 ResponseTerminologyTranslationSvc theResponseTerminologyTranslationSvc) { 056 super(theValidationSupport); 057 myResponseTerminologyTranslationSvc = theResponseTerminologyTranslationSvc; 058 } 059 060 /** 061 * Adds a mapping specification using only a source and target CodeSystem URL. Any mappings specified using 062 * this URL 063 * 064 * @param theSourceCodeSystemUrl The source CodeSystem URL 065 * @param theTargetCodeSystemUrl The target CodeSystem URL 066 */ 067 public void addMappingSpecification(String theSourceCodeSystemUrl, String theTargetCodeSystemUrl) { 068 myResponseTerminologyTranslationSvc.addMappingSpecification(theSourceCodeSystemUrl, theTargetCodeSystemUrl); 069 } 070 071 /** 072 * Clear all mapping specifications 073 */ 074 public void clearMappingSpecifications() { 075 myResponseTerminologyTranslationSvc.clearMappingSpecifications(); 076 } 077 078 public Map<String, String> getMappingSpecifications() { 079 return myResponseTerminologyTranslationSvc.getMappingSpecifications(); 080 } 081 082 @Hook(value = Pointcut.SERVER_OUTGOING_RESPONSE, order = RESPONSE_TERMINOLOGY_TRANSLATION_INTERCEPTOR) 083 public void handleResource(RequestDetails theRequestDetails, IBaseResource theResource) { 084 List<IBaseResource> resources = toListForProcessing(theRequestDetails, theResource); 085 myResponseTerminologyTranslationSvc.processResourcesForTerminologyTranslation(resources); 086 } 087}