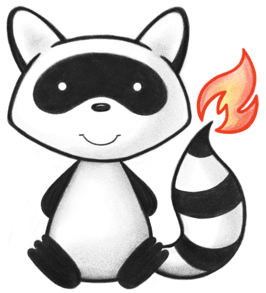
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.interceptor.api.Hook; 023import ca.uhn.fhir.interceptor.api.Pointcut; 024import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import ca.uhn.fhir.rest.server.exceptions.UnprocessableEntityException; 027import ca.uhn.fhir.validation.FhirValidator; 028import ca.uhn.fhir.validation.ResultSeverityEnum; 029import ca.uhn.fhir.validation.ValidationResult; 030import org.apache.commons.lang3.Validate; 031import org.hl7.fhir.instance.model.api.IBaseResource; 032 033import java.util.HashSet; 034import java.util.Set; 035 036/** 037 * This interceptor intercepts each outgoing response and if it contains a FHIR resource, validates that resource. The interceptor may be configured to run any validator modules, and will then add 038 * headers to the response or fail the request with an {@link UnprocessableEntityException HTTP 422 Unprocessable Entity}. 039 */ 040public class ResponseValidatingInterceptor extends BaseValidatingInterceptor<IBaseResource> { 041 042 /** 043 * X-HAPI-Request-Validation 044 */ 045 public static final String DEFAULT_RESPONSE_HEADER_NAME = "X-FHIR-Response-Validation"; 046 047 private static final org.slf4j.Logger ourLog = 048 org.slf4j.LoggerFactory.getLogger(ResponseValidatingInterceptor.class); 049 050 private Set<RestOperationTypeEnum> myExcludeOperationTypes; 051 052 /** 053 * Do not validate the following operations. A common use for this is to exclude {@link RestOperationTypeEnum#METADATA} so that this operation will execute as quickly as possible. 054 */ 055 public void addExcludeOperationType(RestOperationTypeEnum theOperationType) { 056 Validate.notNull(theOperationType, "theOperationType must not be null"); 057 if (myExcludeOperationTypes == null) { 058 myExcludeOperationTypes = new HashSet<>(); 059 } 060 myExcludeOperationTypes.add(theOperationType); 061 } 062 063 @Override 064 ValidationResult doValidate(FhirValidator theValidator, IBaseResource theRequest) { 065 return theValidator.validateWithResult(theRequest); 066 } 067 068 @Hook(Pointcut.SERVER_OUTGOING_RESPONSE) 069 public boolean outgoingResponse(RequestDetails theRequestDetails, IBaseResource theResponseObject) { 070 RestOperationTypeEnum operationType = theRequestDetails.getRestOperationType(); 071 if (operationType != null 072 && myExcludeOperationTypes != null 073 && myExcludeOperationTypes.contains(operationType)) { 074 ourLog.trace("Operation type {} is excluded from validation", operationType); 075 return true; 076 } 077 078 validate(theResponseObject, theRequestDetails); 079 080 return true; 081 } 082 083 @Override 084 String provideDefaultResponseHeaderName() { 085 return DEFAULT_RESPONSE_HEADER_NAME; 086 } 087 088 /** 089 * Sets the name of the response header to add validation failures to 090 * 091 * @see #DEFAULT_RESPONSE_HEADER_NAME 092 * @see #setAddResponseHeaderOnSeverity(ResultSeverityEnum) 093 */ 094 @Override 095 public void setResponseHeaderName(String theResponseHeaderName) { 096 super.setResponseHeaderName(theResponseHeaderName); 097 } 098}