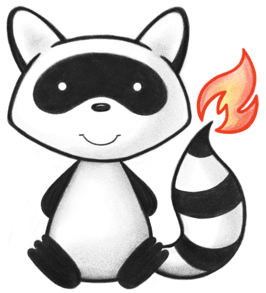
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.interceptor.api.HookParams; 023import ca.uhn.fhir.interceptor.api.IInterceptorBroadcaster; 024import ca.uhn.fhir.interceptor.api.Pointcut; 025import ca.uhn.fhir.rest.api.server.IPreResourceShowDetails; 026import ca.uhn.fhir.rest.api.server.RequestDetails; 027import ca.uhn.fhir.rest.api.server.SimplePreResourceShowDetails; 028import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 029import ca.uhn.fhir.rest.server.util.CompositeInterceptorBroadcaster; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031 032import java.util.List; 033import java.util.Objects; 034import javax.annotation.CheckReturnValue; 035 036public class ServerInterceptorUtil { 037 038 private ServerInterceptorUtil() { 039 super(); 040 } 041 042 /** 043 * Fires {@link Pointcut#STORAGE_PRESHOW_RESOURCES} interceptor hook, and potentially remove resources 044 * from the resource list 045 */ 046 @CheckReturnValue 047 public static List<IBaseResource> fireStoragePreshowResource( 048 List<IBaseResource> theResources, 049 RequestDetails theRequest, 050 IInterceptorBroadcaster theInterceptorBroadcaster) { 051 List<IBaseResource> retVal = theResources; 052 retVal.removeIf(Objects::isNull); 053 054 // Interceptor call: STORAGE_PRESHOW_RESOURCE 055 // This can be used to remove results from the search result details before 056 // the user has a chance to know that they were in the results 057 if (!retVal.isEmpty()) { 058 IInterceptorBroadcaster compositeBroadcaster = 059 CompositeInterceptorBroadcaster.newCompositeBroadcaster(theInterceptorBroadcaster, theRequest); 060 if (compositeBroadcaster.hasHooks(Pointcut.STORAGE_PRESHOW_RESOURCES)) { 061 SimplePreResourceShowDetails accessDetails = new SimplePreResourceShowDetails(retVal); 062 HookParams params = new HookParams() 063 .add(IPreResourceShowDetails.class, accessDetails) 064 .add(RequestDetails.class, theRequest) 065 .addIfMatchesType(ServletRequestDetails.class, theRequest); 066 compositeBroadcaster.callHooks(Pointcut.STORAGE_PRESHOW_RESOURCES, params); 067 068 retVal = accessDetails.toList(); 069 retVal.removeIf(Objects::isNull); 070 } 071 } 072 073 return retVal; 074 } 075}