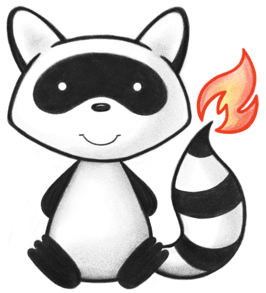
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.interceptor.api.Hook; 023import ca.uhn.fhir.interceptor.api.Interceptor; 024import ca.uhn.fhir.interceptor.api.Pointcut; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import ca.uhn.fhir.validation.ValidationResult; 027import jakarta.annotation.Nonnull; 028import jakarta.annotation.Nullable; 029import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031 032import java.util.ArrayList; 033import java.util.List; 034 035@Interceptor 036public class ValidationResultEnrichingInterceptor { 037 038 /** 039 * A {@link RequestDetails#getUserData() user data} entry will be created with this 040 * key which contains the {@link ValidationResult} from validating the request. 041 */ 042 public static final String REQUEST_VALIDATION_RESULT = 043 ValidationResultEnrichingInterceptor.class.getName() + "_REQUEST_VALIDATION_RESULT"; 044 045 @SuppressWarnings("unchecked") 046 @Hook(Pointcut.SERVER_OUTGOING_RESPONSE) 047 public boolean addValidationResultsToOperationOutcome( 048 RequestDetails theRequestDetails, IBaseResource theResponseObject) { 049 if (theResponseObject instanceof IBaseOperationOutcome) { 050 IBaseOperationOutcome oo = (IBaseOperationOutcome) theResponseObject; 051 052 if (theRequestDetails != null) { 053 List<ValidationResult> validationResult = 054 (List<ValidationResult>) theRequestDetails.getUserData().remove(REQUEST_VALIDATION_RESULT); 055 if (validationResult != null) { 056 for (ValidationResult next : validationResult) { 057 next.populateOperationOutcome(oo); 058 } 059 } 060 } 061 } 062 063 return true; 064 } 065 066 @SuppressWarnings("unchecked") 067 public static void addValidationResultToRequestDetails( 068 @Nullable RequestDetails theRequestDetails, @Nonnull ValidationResult theValidationResult) { 069 if (theRequestDetails != null) { 070 List<ValidationResult> results = (List<ValidationResult>) 071 theRequestDetails.getUserData().computeIfAbsent(REQUEST_VALIDATION_RESULT, t -> new ArrayList<>(2)); 072 results.add(theValidationResult); 073 } 074 } 075}