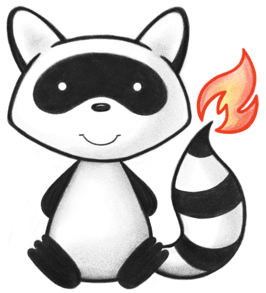
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor; 021 022import ca.uhn.fhir.rest.api.server.RequestDetails; 023import ca.uhn.fhir.rest.server.exceptions.AuthenticationException; 024import jakarta.servlet.http.HttpServletRequest; 025import jakarta.servlet.http.HttpServletResponse; 026 027import java.util.Enumeration; 028 029/** 030 * This interceptor creates verbose server log entries containing the complete request and response payloads. 031 * <p> 032 * This interceptor is mainly intended for debugging since it will generate very large log entries and 033 * could potentially be a security risk since it logs every header and complete payload. Use with caution! 034 * </p> 035 */ 036public class VerboseLoggingInterceptor extends InterceptorAdapter { 037 038 private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(VerboseLoggingInterceptor.class); 039 040 @Override 041 public boolean incomingRequestPostProcessed( 042 RequestDetails theRequestDetails, HttpServletRequest theRequest, HttpServletResponse theResponse) 043 throws AuthenticationException { 044 045 StringBuilder b = new StringBuilder("Incoming request: "); 046 b.append(theRequest.getMethod()); 047 b.append(" "); 048 b.append(theRequest.getRequestURL()); 049 b.append("\n"); 050 051 for (Enumeration<String> headerEnumeration = theRequest.getHeaderNames(); 052 headerEnumeration.hasMoreElements(); ) { 053 String nextName = headerEnumeration.nextElement(); 054 for (Enumeration<String> valueEnumeration = theRequest.getHeaders(nextName); 055 valueEnumeration.hasMoreElements(); ) { 056 b.append(" * ") 057 .append(nextName) 058 .append(": ") 059 .append(valueEnumeration.nextElement()) 060 .append("\n"); 061 } 062 } 063 064 ourLog.info(b.toString()); 065 return true; 066 } 067}