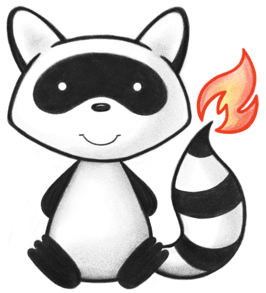
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import ca.uhn.fhir.interceptor.api.Pointcut; 023import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.server.interceptor.auth.AuthorizationInterceptor.Verdict; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.hl7.fhir.instance.model.api.IIdType; 028 029import java.util.Set; 030 031/** 032 * Note: At this time, this interface is considered internal API to HAPI FHIR, 033 * and is subject to change without warning. Create your own implementations at 034 * your own risk. If you have use cases that are not met by the current 035 * implementation, please consider raising them on the HAPI FHIR 036 * Google Group. 037 */ 038public interface IAuthRule { 039 040 /** 041 * Applies the rule and returns a policy decision, or <code>null</code> if the rule does not apply 042 * 043 * @param theOperation The operation type 044 * @param theRequestDetails The request 045 * @param theInputResource The resource being input by the client, or <code>null</code> 046 * @param theInputResourceId TODO 047 * @param theOutputResource The resource being returned by the server, or <code>null</code> 048 * @param theRuleApplier The rule applying module (this can be used by rules to apply the rule set to 049 * nested objects in the request, such as nested requests in a transaction) 050 * @param theFlags The flags configured in the authorization interceptor 051 * @param thePointcut The pointcut hook that triggered this call 052 * @return Returns a policy decision, or <code>null</code> if the rule does not apply 053 */ 054 Verdict applyRule( 055 RestOperationTypeEnum theOperation, 056 RequestDetails theRequestDetails, 057 IBaseResource theInputResource, 058 IIdType theInputResourceId, 059 IBaseResource theOutputResource, 060 IRuleApplier theRuleApplier, 061 Set<AuthorizationFlagsEnum> theFlags, 062 Pointcut thePointcut); 063 064 /** 065 * Returns a name for this rule, to be used in logs and error messages 066 */ 067 String getName(); 068}