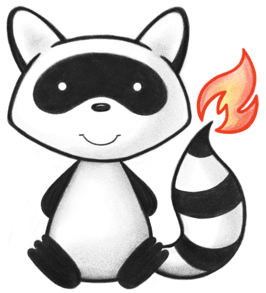
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import java.util.List; 023 024/** 025 * Used by {@link AuthorizationInterceptor} in order to allow user code to define authorization 026 * rules. 027 * 028 * @see AuthorizationInterceptor 029 */ 030public interface IAuthRuleBuilder { 031 032 /** 033 * Start a new rule to allow a given operation 034 */ 035 IAuthRuleBuilderRule allow(); 036 037 /** 038 * Start a new rule to allow a given operation 039 * 040 * @param theRuleName 041 * The name of this rule. The rule name is used for logging and error messages, 042 * and could be shown to the client, but has no semantic meaning within 043 * HAPI FHIR. 044 */ 045 IAuthRuleBuilderRule allow(String theRuleName); 046 047 /** 048 * This rule allows any invocation to proceed. It is intended to be 049 * used at the end of a chain that contains {@link #deny()} rules in 050 * order to specify a blacklist chain. 051 * <p> 052 * This call completes the rule and adds the rule to the chain. 053 * </p> 054 */ 055 IAuthRuleBuilderRuleOpClassifierFinished allowAll(); 056 057 /** 058 * This rule allows any invocation to proceed. It is intended to be 059 * used at the end of a chain that contains {@link #deny()} rules in 060 * order to specify a blacklist chain. 061 * <p> 062 * This call completes the rule and adds the rule to the chain. 063 * </p> 064 * @param theRuleName 065 * The name of this rule. The rule name is used for logging and error messages, 066 * and could be shown to the client, but has no semantic meaning within 067 * HAPI FHIR. 068 */ 069 IAuthRuleBuilderRuleOpClassifierFinished allowAll(String theRuleName); 070 071 /** 072 * Build the rule list 073 */ 074 List<IAuthRule> build(); 075 076 /** 077 * Start a new rule to deny a given operation 078 */ 079 IAuthRuleBuilderRule deny(); 080 081 /** 082 * Start a new rule to deny a given operation 083 * 084 * @param theRuleName 085 * The name of this rule. The rule name is used for logging and error messages, 086 * and could be shown to the client, but has no semantic meaning within 087 * HAPI FHIR. 088 */ 089 IAuthRuleBuilderRule deny(String theRuleName); 090 091 /** 092 * This rule allows any invocation to proceed. It is intended to be 093 * used at the end of a chain that contains {@link #allow()} rules in 094 * order to specify a whitelist chain. 095 * <p> 096 * This call completes the rule and adds the rule to the chain. 097 * </p> 098 */ 099 IAuthRuleBuilderRuleOpClassifierFinished denyAll(); 100 101 /** 102 * This rule allows any invocation to proceed. It is intended to be 103 * used at the end of a chain that contains {@link #allow()} rules in 104 * order to specify a whitelist chain. 105 * <p> 106 * This call completes the rule and adds the rule to the chain. 107 * </p> 108 * @param theRuleName 109 * The name of this rule. The rule name is used for logging and error messages, 110 * and could be shown to the client, but has no semantic meaning within 111 * HAPI FHIR. 112 */ 113 IAuthRuleBuilderRuleOpClassifierFinished denyAll(String theRuleName); 114}