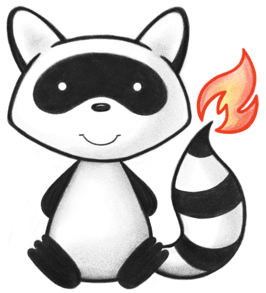
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import org.hl7.fhir.instance.model.api.IIdType; 023 024import java.util.Collection; 025 026public interface IAuthRuleBuilderRuleOp extends IAuthRuleBuilderAppliesTo<IAuthRuleBuilderRuleOpClassifier> { 027 028 /** 029 * Rule applies to the resource with the given ID (e.g. <code>Patient/123</code>) 030 * <p> 031 * See the following examples which show how theId is interpreted: 032 * </p> 033 * <ul> 034 * <li><b><code>http://example.com/Patient/123</code></b> - Any Patient resource with the ID "123" will be matched (note: the base URL part is ignored)</li> 035 * <li><b><code>Patient/123</code></b> - Any Patient resource with the ID "123" will be matched</li> 036 * <li><b><code>123</code></b> - Any resource of any type with the ID "123" will be matched</li> 037 * </ul> 038 * 039 * @param theId The ID of the resource to apply (e.g. <code>Patient/123</code>) 040 * @throws IllegalArgumentException If theId does not contain an ID with at least an ID part 041 * @throws NullPointerException If theId is null 042 */ 043 IAuthRuleFinished instance(String theId); 044 045 /** 046 * Rule applies to the resource with the given ID (e.g. <code>Patient/123</code>) 047 * <p> 048 * See the following examples which show how theId is interpreted: 049 * </p> 050 * <ul> 051 * <li><b><code>http://example.com/Patient/123</code></b> - Any Patient resource with the ID "123" will be matched (note: the base URL part is ignored)</li> 052 * <li><b><code>Patient/123</code></b> - Any Patient resource with the ID "123" will be matched</li> 053 * <li><b><code>123</code></b> - Any resource of any type with the ID "123" will be matched</li> 054 * </ul> 055 * >* 056 * @param theId The ID of the resource to apply (e.g. <code>Patient/123</code>) 057 * @throws IllegalArgumentException If theId does not contain an ID with at least an ID part 058 * @throws NullPointerException If theId is null 059 */ 060 IAuthRuleFinished instance(IIdType theId); 061 062 /** 063 * Rule applies to the resource with the given ID (e.g. <code>Patient/123</code>) 064 * <p> 065 * See the following examples which show how theId is interpreted: 066 * </p> 067 * <ul> 068 * <li><b><code>http://example.com/Patient/123</code></b> - Any Patient resource with the ID "123" will be matched (note: the base URL part is ignored)</li> 069 * <li><b><code>Patient/123</code></b> - Any Patient resource with the ID "123" will be matched</li> 070 * <li><b><code>123</code></b> - Any resource of any type with the ID "123" will be matched</li> 071 * </ul> 072 * 073 * @param theIds The IDs of the resource to apply (e.g. <code>Patient/123</code>) 074 * @throws IllegalArgumentException If theId does not contain an ID with at least an ID part 075 * @throws NullPointerException If theId is null 076 */ 077 IAuthRuleFinished instances(Collection<IIdType> theIds); 078}