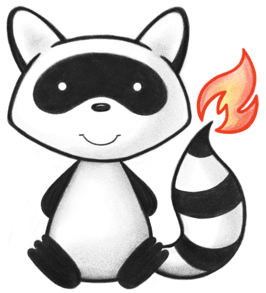
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import jakarta.annotation.Nonnull; 023import org.hl7.fhir.instance.model.api.IIdType; 024 025import java.util.Collection; 026 027public interface IAuthRuleBuilderRuleOpClassifier { 028 029 /** 030 * Rule applies to resources in the given compartment. 031 * <p> 032 * For example, to apply the rule to any observations in the patient compartment 033 * belonging to patient "123", you would invoke this with</br> 034 * <code>inCompartment("Patient", new IdType("Patient", "123"))</code> 035 * </p> 036 * <p> 037 * This call completes the rule and adds the rule to the chain. 038 * </p> 039 * 040 * @param theCompartmentName The name of the compartment (must not be null or blank) 041 * @param theOwner The owner of the compartment. Note that both the resource type and ID must be populated in this ID. 042 */ 043 IAuthRuleBuilderRuleOpClassifierFinished inCompartment(String theCompartmentName, IIdType theOwner); 044 045 /** 046 * Rule applies to resources in the given compartment. 047 * <p> 048 * For example, to apply the rule to any observations in the patient compartment 049 * belonging to patient "123", you would invoke this with</br> 050 * <code>inCompartment("Patient", new IdType("Patient", "123"))</code> 051 * 052 * This call also allows you to pass additional search parameters that count as being included in the given compartment, 053 * passed in as a list of `resourceType:search-parameter-name`. For example, if you select a compartment name of "patient", 054 * you could pass in a singleton list consisting of the string "device:patient", which would cause any devices belonging 055 * to the patient to be permitted by the authorization rule. 056 * 057 * </p> 058 * <p> 059 * This call completes the rule and adds the rule to the chain. 060 * </p> 061 * 062 * @param theCompartmentName The name of the compartment (must not be null or blank) 063 * @param theOwner The owner of the compartment. Note that both the resource type and ID must be populated in this ID. 064 * @param theAdditionalTypeSearchParamNames A list of strings for additional resource types and search parameters which count as being in the compartment, in the form "resourcetype:search-parameter-name". 065 */ 066 IAuthRuleBuilderRuleOpClassifierFinished inCompartmentWithAdditionalSearchParams( 067 String theCompartmentName, 068 IIdType theOwner, 069 AdditionalCompartmentSearchParameters theAdditionalTypeSearchParamNames); 070 071 /** 072 * Rule applies to resources in the given compartment. 073 * <p> 074 * For example, to apply the rule to any observations in the patient compartment 075 * belonging to patient "123", you would invoke this with</br> 076 * <code>inCompartment("Patient", new IdType("Patient", "123"))</code> 077 * </p> 078 * <p> 079 * This call completes the rule and adds the rule to the chain. 080 * </p> 081 * 082 * @param theCompartmentName The name of the compartment (must not be null or blank) 083 * @param theOwners The owner of the compartment. Note that both the resource type and ID must be populated in this ID. 084 */ 085 IAuthRuleBuilderRuleOpClassifierFinished inCompartment( 086 String theCompartmentName, Collection<? extends IIdType> theOwners); 087 088 /** 089 * Rule applies to resources in the given compartment. 090 * <p> 091 * For example, to apply the rule to any observations in the patient compartment 092 * belonging to patient "123", you would invoke this with</br> 093 * <code>inCompartment("Patient", new IdType("Patient", "123"))</code> 094 * 095 * This call also allows you to pass additional search parameters that count as being included in the given compartment, 096 * passed in as a list of `resourceType:search-parameter-name`. For example, if you select a compartment name of "patient", 097 * you could pass in a singleton list consisting of the string "device:patient", which would cause any devices belonging 098 * to the patient to be permitted by the authorization rule. 099 * 100 * </p> 101 * <p> 102 * This call completes the rule and adds the rule to the chain. 103 * </p> 104 * 105 * @param theCompartmentName The name of the compartment (must not be null or blank) 106 * @param theOwners The owners of the compartment. Note that both the resource type and ID must be populated in these IDs. 107 * @param theAdditionalTypeSearchParamNames A {@link AdditionalCompartmentSearchParameters} which allows you to expand the search space for what is considered "in" the compartment. 108 * 109 **/ 110 IAuthRuleBuilderRuleOpClassifierFinished inCompartmentWithAdditionalSearchParams( 111 String theCompartmentName, 112 Collection<? extends IIdType> theOwners, 113 AdditionalCompartmentSearchParameters theAdditionalTypeSearchParamNames); 114 115 /** 116 * Rule applies to any resource instances 117 * <p> 118 * This call completes the rule and adds the rule to the chain. 119 * </p> 120 */ 121 IAuthRuleBuilderRuleOpClassifierFinished withAnyId(); 122 123 /** 124 * Rule applies to resources where the given search parameter would be satisfied by a code in the given ValueSet 125 * @param theSearchParameterName The search parameter name, e.g. <code>"code"</code> 126 * @param theValueSetUrl The valueset URL, e.g. <code>"http://my-value-set"</code> 127 * @since 6.0.0 128 */ 129 IAuthRuleBuilderRuleOpClassifierFinished withCodeInValueSet( 130 @Nonnull String theSearchParameterName, @Nonnull String theValueSetUrl); 131 132 /** 133 * Rule applies to resources where the given search parameter would be satisfied by a code not in the given ValueSet 134 * @param theSearchParameterName The search parameter name, e.g. <code>"code"</code> 135 * @param theValueSetUrl The valueset URL, e.g. <code>"http://my-value-set"</code> 136 * @since 6.0.0 137 */ 138 IAuthRuleFinished withCodeNotInValueSet(@Nonnull String theSearchParameterName, @Nonnull String theValueSetUrl); 139 140 IAuthRuleFinished inCompartmentWithFilter(String theCompartment, IIdType theIdElement, String theFilter); 141 142 IAuthRuleFinished withFilter(String theFilter); 143}