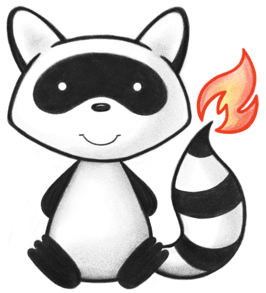
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import jakarta.annotation.Nonnull; 023import jakarta.annotation.Nullable; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026/** 027 * Adapt the InMemoryMatcher to support authorization filters in {@link FhirQueryRuleTester}. 028 * Exists because filters may be applied to resources that don't support all paramters, and UNSUPPORTED 029 * has a different meaning during authorization. 030 */ 031public interface IAuthorizationSearchParamMatcher { 032 /** 033 * Calculate if the resource would match the fhir query parameters. 034 * @param theQueryParameters e.g. "category=laboratory" 035 * @param theResource the target of the comparison 036 */ 037 MatchResult match(String theQueryParameters, IBaseResource theResource); 038 039 /** 040 * Match outcomes. 041 */ 042 enum Match { 043 MATCH, 044 NO_MATCH, 045 /** Used for contexts without matcher infrastructure like hybrid providers */ 046 UNSUPPORTED 047 } 048 049 class MatchResult { 050 // fake record pattern 051 /** match result */ 052 @Nonnull 053 public final Match match; 054 /** the reason for the UNSUPPORTED result */ 055 @Nullable 056 public final String unsupportedReason; 057 058 public static MatchResult buildMatched() { 059 return new MatchResult(Match.MATCH, null); 060 } 061 062 public static MatchResult buildUnmatched() { 063 return new MatchResult(Match.NO_MATCH, null); 064 } 065 066 public static MatchResult buildUnsupported(@Nonnull String theReason) { 067 return new MatchResult(Match.UNSUPPORTED, theReason); 068 } 069 070 private MatchResult(Match myMatch, String myUnsupportedReason) { 071 this.match = myMatch; 072 this.unsupportedReason = myUnsupportedReason; 073 } 074 } 075}