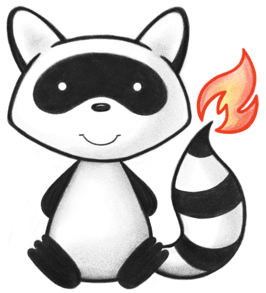
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import ca.uhn.fhir.interceptor.api.Pointcut; 023import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.rest.server.interceptor.auth.AuthorizationInterceptor.Verdict; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.hl7.fhir.instance.model.api.IIdType; 028 029import java.util.Set; 030 031public class RuleImplConditional extends BaseRule implements IAuthRule { 032 033 private AppliesTypeEnum myAppliesTo; 034 private Set<String> myAppliesToTypes; 035 private RestOperationTypeEnum myOperationType; 036 037 RuleImplConditional(String theRuleName) { 038 super(theRuleName); 039 } 040 041 @Override 042 public Verdict applyRule( 043 RestOperationTypeEnum theOperation, 044 RequestDetails theRequestDetails, 045 IBaseResource theInputResource, 046 IIdType theInputResourceId, 047 IBaseResource theOutputResource, 048 IRuleApplier theRuleApplier, 049 Set<AuthorizationFlagsEnum> theFlags, 050 Pointcut thePointcut) { 051 assert !(theInputResource != null && theOutputResource != null); 052 053 if (theInputResourceId != null && theInputResourceId.hasIdPart()) { 054 return null; 055 } 056 057 if (theOperation == myOperationType) { 058 if (theRequestDetails.getConditionalUrl(myOperationType) == null) { 059 return null; 060 } 061 if (theInputResource == null) { 062 return null; 063 } 064 065 switch (myAppliesTo) { 066 case ALL_RESOURCES: 067 case INSTANCES: 068 break; 069 case TYPES: 070 if (myOperationType == RestOperationTypeEnum.DELETE) { 071 String resourceName = theRequestDetails.getResourceName(); 072 if (!myAppliesToTypes.contains(resourceName)) { 073 return null; 074 } 075 } else { 076 String inputResourceName = 077 theRequestDetails.getFhirContext().getResourceType(theInputResource); 078 if (!myAppliesToTypes.contains(inputResourceName)) { 079 return null; 080 } 081 } 082 break; 083 } 084 085 return newVerdict( 086 theOperation, 087 theRequestDetails, 088 theInputResource, 089 theInputResourceId, 090 theOutputResource, 091 theRuleApplier); 092 } 093 094 return null; 095 } 096 097 void setAppliesTo(AppliesTypeEnum theAppliesTo) { 098 myAppliesTo = theAppliesTo; 099 } 100 101 void setAppliesToTypes(Set<String> theAppliesToTypes) { 102 myAppliesToTypes = theAppliesToTypes; 103 } 104 105 void setOperationType(RestOperationTypeEnum theOperationType) { 106 myOperationType = theOperationType; 107 } 108}