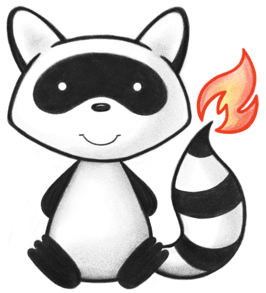
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import ca.uhn.fhir.interceptor.api.Pointcut; 023import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import org.hl7.fhir.instance.model.api.IBaseResource; 026import org.hl7.fhir.instance.model.api.IIdType; 027 028import java.util.Set; 029 030public class RuleImplUpdateHistoryRewrite extends BaseRule { 031 032 private boolean myAllRequests; 033 034 RuleImplUpdateHistoryRewrite(String theRuleName) { 035 super(theRuleName); 036 } 037 038 @Override 039 public AuthorizationInterceptor.Verdict applyRule( 040 RestOperationTypeEnum theOperation, 041 RequestDetails theRequestDetails, 042 IBaseResource theInputResource, 043 IIdType theInputResourceId, 044 IBaseResource theOutputResource, 045 IRuleApplier theRuleApplier, 046 Set<AuthorizationFlagsEnum> theFlags, 047 Pointcut thePointcut) { 048 if (myAllRequests) { 049 if (theRequestDetails.getId() != null 050 && theRequestDetails.getId().hasVersionIdPart() 051 && theOperation == RestOperationTypeEnum.UPDATE) { 052 return newVerdict( 053 theOperation, 054 theRequestDetails, 055 theInputResource, 056 theInputResourceId, 057 theOutputResource, 058 theRuleApplier); 059 } 060 } 061 062 return null; 063 } 064 065 RuleImplUpdateHistoryRewrite setAllRequests(boolean theAllRequests) { 066 myAllRequests = theAllRequests; 067 return this; 068 } 069}