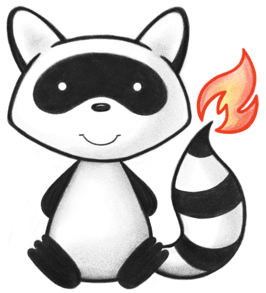
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.rest.api.server.RequestDetails; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025import org.hl7.fhir.instance.model.api.IIdType; 026 027import java.util.Collection; 028import java.util.HashMap; 029import java.util.Map; 030 031public class RuleTarget { 032 IBaseResource resource; 033 Collection<IIdType> resourceIds = null; 034 String resourceType = null; 035 private Map<String, String[]> mySearchParams = null; 036 037 public Map<String, String[]> getSearchParams() { 038 return mySearchParams; 039 } 040 041 public void setSearchParams(RequestDetails theRequestDetails) { 042 mySearchParams = stripMdmSuffix(theRequestDetails.getParameters()); 043 } 044 045 private Map<String, String[]> stripMdmSuffix(Map<String, String[]> theParameters) { 046 Map<String, String[]> retval = new HashMap<>(); 047 for (Map.Entry<String, String[]> entry : theParameters.entrySet()) { 048 String key = entry.getKey(); 049 String[] value = entry.getValue(); 050 key = stripMdmQualifier(key); 051 key = stripNicknameQualifier(key); 052 retval.put(key, value); 053 } 054 return retval; 055 } 056 057 private String stripMdmQualifier(String theKey) { 058 if (theKey.endsWith(Constants.PARAMQUALIFIER_MDM)) { 059 theKey = theKey.split(Constants.PARAMQUALIFIER_MDM)[0]; 060 } 061 return theKey; 062 } 063 064 private String stripNicknameQualifier(String theKey) { 065 if (theKey.endsWith(Constants.PARAMQUALIFIER_NICKNAME)) { 066 theKey = theKey.split(Constants.PARAMQUALIFIER_NICKNAME)[0]; 067 } 068 return theKey; 069 } 070}