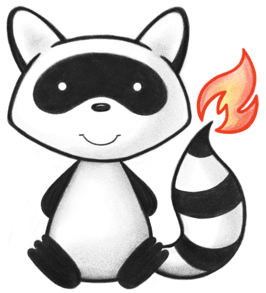
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.s13n.standardizers; 021 022import org.apache.commons.text.WordUtils; 023 024import java.util.Arrays; 025import java.util.HashSet; 026import java.util.Set; 027 028/** 029 * Standardizes last names by capitalizing all characters following a separators (e.g. -, '), capitalizing "Mac" and "Mc" 030 * prefixes and keeping name particles in lower case. 031 */ 032public class LastNameStandardizer extends FirstNameStandardizer { 033 034 private Set<String> myParticles = new HashSet<>(Arrays.asList("van", "der", "ter", "de", "da", "la")); 035 private Set<String> myPrefixes = new HashSet<>(Arrays.asList("mac", "mc")); 036 private Set<String> myPrefixExcludes = new HashSet<>(Arrays.asList("machi")); 037 038 public LastNameStandardizer() { 039 super(); 040 } 041 042 protected LastNameStandardizer addDelimiters(String... theDelimiters) { 043 super.addDelimiters(theDelimiters); 044 return this; 045 } 046 047 protected String standardizeNameToken(String theToken) { 048 if (theToken.isEmpty()) { 049 return theToken; 050 } 051 052 if (myParticles.contains(theToken.toLowerCase())) { 053 return theToken.toLowerCase(); 054 } 055 056 String retVal = super.standardizeNameToken(theToken); 057 return handlePrefix(retVal); 058 } 059 060 protected String handlePrefix(String theToken) { 061 String lowerCaseToken = theToken.toLowerCase(); 062 for (String exclude : myPrefixExcludes) { 063 if (lowerCaseToken.startsWith(exclude)) { 064 return theToken; 065 } 066 } 067 068 for (String prefix : myPrefixes) { 069 if (!lowerCaseToken.startsWith(prefix)) { 070 continue; 071 } 072 073 String capitalizedPrefix = WordUtils.capitalize(prefix); 074 String capitalizedSuffix = WordUtils.capitalize(lowerCaseToken.replaceFirst(prefix, "")); 075 return capitalizedPrefix.concat(capitalizedSuffix); 076 } 077 return theToken; 078 } 079}