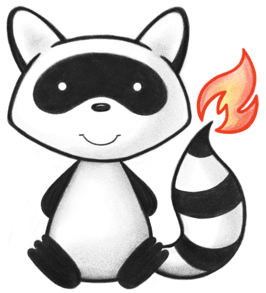
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.validation.address; 021 022import org.hl7.fhir.instance.model.api.IBase; 023 024import java.util.HashMap; 025import java.util.Map; 026 027public class AddressValidationResult { 028 029 private boolean myIsValid; 030 private String myValidatedAddressString; 031 private Map<String, String> myValidationResults = new HashMap<>(); 032 private String myRawResponse; 033 private IBase myValidatedAddress; 034 035 public boolean isValid() { 036 return myIsValid; 037 } 038 039 public void setValid(boolean theIsValid) { 040 this.myIsValid = theIsValid; 041 } 042 043 public Map<String, String> getValidationResults() { 044 return myValidationResults; 045 } 046 047 public void setValidationResults(Map<String, String> myValidationResults) { 048 this.myValidationResults = myValidationResults; 049 } 050 051 public String getValidatedAddressString() { 052 return myValidatedAddressString; 053 } 054 055 public void setValidatedAddressString(String theValidatedAddressString) { 056 this.myValidatedAddressString = theValidatedAddressString; 057 } 058 059 public IBase getValidatedAddress() { 060 return myValidatedAddress; 061 } 062 063 public void setValidatedAddress(IBase theValidatedAddress) { 064 this.myValidatedAddress = theValidatedAddress; 065 } 066 067 public String getRawResponse() { 068 return myRawResponse; 069 } 070 071 public void setRawResponse(String theRawResponse) { 072 this.myRawResponse = theRawResponse; 073 } 074 075 @Override 076 public String toString() { 077 return " isValid=" + myIsValid + ", validatedAddressString='" 078 + myValidatedAddressString + '\'' + ", validationResults=" 079 + myValidationResults + '\'' + ", rawResponse='" 080 + myRawResponse + '\'' + ", myValidatedAddress='" 081 + myValidatedAddress + '\''; 082 } 083}