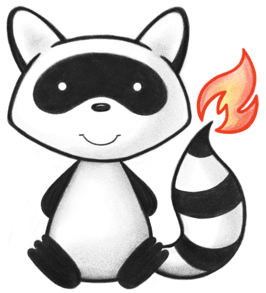
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.validation.helpers; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.util.PropertyModifyingHelper; 024import org.apache.commons.lang3.StringUtils; 025import org.hl7.fhir.instance.model.api.IBase; 026 027import java.util.Arrays; 028import java.util.List; 029import java.util.stream.Collectors; 030 031/** 032 * Helper class for working with FHIR Address element 033 */ 034public class AddressHelper extends PropertyModifyingHelper { 035 036 public static final String FIELD_LINE = "line"; 037 public static final String FIELD_CITY = "city"; 038 public static final String FIELD_TEXT = "text"; 039 public static final String FIELD_DISTRICT = "district"; 040 public static final String FIELD_STATE = "state"; 041 public static final String FIELD_POSTAL = "postalCode"; 042 public static final String FIELD_COUNTRY = "country"; 043 044 public static final String[] FIELD_NAMES = { 045 FIELD_TEXT, FIELD_LINE, FIELD_CITY, FIELD_DISTRICT, FIELD_STATE, FIELD_POSTAL, FIELD_COUNTRY 046 }; 047 048 public static final String[] ADDRESS_PARTS = {FIELD_CITY, FIELD_DISTRICT, FIELD_STATE, FIELD_POSTAL}; 049 050 public AddressHelper(FhirContext theFhirContext, IBase theBase) { 051 super(theFhirContext, theBase); 052 } 053 054 public String getCountry() { 055 return get(FIELD_COUNTRY); 056 } 057 058 public String getCity() { 059 return get(FIELD_CITY); 060 } 061 062 public String getState() { 063 return get(FIELD_STATE); 064 } 065 066 public String getPostalCode() { 067 return get(FIELD_POSTAL); 068 } 069 070 public String getText() { 071 return get(FIELD_TEXT); 072 } 073 074 public void setCountry(String theCountry) { 075 set(FIELD_COUNTRY, theCountry); 076 } 077 078 public void setCity(String theCity) { 079 set(FIELD_CITY, theCity); 080 } 081 082 public void setState(String theState) { 083 set(FIELD_STATE, theState); 084 } 085 086 public void setPostalCode(String thePostal) { 087 set(FIELD_POSTAL, thePostal); 088 } 089 090 public void setText(String theText) { 091 set(FIELD_TEXT, theText); 092 } 093 094 public String getParts() { 095 return Arrays.stream(ADDRESS_PARTS) 096 .map(this::get) 097 .filter(s -> !StringUtils.isBlank(s)) 098 .collect(Collectors.joining(getDelimiter())); 099 } 100 101 public String getLine() { 102 return get(FIELD_LINE); 103 } 104 105 public List<String> getLines() { 106 return getMultiple(FIELD_LINE); 107 } 108 109 public AddressHelper addLine(String theLine) { 110 set(FIELD_LINE, theLine); 111 return this; 112 } 113 114 public <T> T getAddress() { 115 return (T) getBase(); 116 } 117 118 @Override 119 public String toString() { 120 return getFields(FIELD_NAMES); 121 } 122}