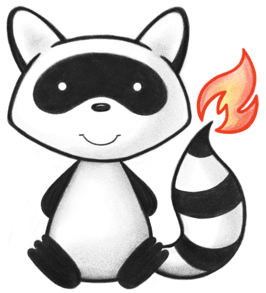
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.messaging.json; 021 022import ca.uhn.fhir.interceptor.model.IDefaultPartitionSettings; 023import ca.uhn.fhir.model.api.IModelJson; 024import ca.uhn.fhir.rest.server.messaging.IHasPayloadMessageKey; 025import ca.uhn.fhir.rest.server.messaging.IMessage; 026import ca.uhn.fhir.rest.server.messaging.IMessageDeliveryContext; 027import ca.uhn.fhir.rest.server.messaging.RequestPartitionHeaderUtil; 028import com.fasterxml.jackson.annotation.JsonProperty; 029import jakarta.annotation.Nonnull; 030import org.springframework.messaging.Message; 031import org.springframework.messaging.MessageHeaders; 032 033import java.util.Map; 034 035import static java.util.Objects.isNull; 036 037public abstract class BaseJsonMessage<T> implements IMessage<T>, Message<T>, IModelJson, IMessageDeliveryContext { 038 039 @JsonProperty("headers") 040 private HapiMessageHeaders myHeaders; 041 042 /** 043 * Constructor 044 */ 045 public BaseJsonMessage() { 046 super(); 047 setDefaultRetryHeaders(); 048 } 049 050 protected void setDefaultRetryHeaders() { 051 HapiMessageHeaders messageHeaders = new HapiMessageHeaders(); 052 setHeaders(messageHeaders); 053 } 054 055 @Override 056 @Nonnull 057 public MessageHeaders getHeaders() { 058 return getHapiHeaders().toMessageHeaders(); 059 } 060 061 public HapiMessageHeaders getHapiHeaders() { 062 if (isNull(myHeaders)) { 063 setDefaultRetryHeaders(); 064 } 065 return myHeaders; 066 } 067 068 public void setHeaders(HapiMessageHeaders theHeaders) { 069 myHeaders = theHeaders; 070 } 071 072 @Override 073 @Nonnull 074 public String getMessageKey() { 075 T payload = getPayload(); 076 if (payload instanceof IHasPayloadMessageKey) { 077 String payloadMessageKey = ((IHasPayloadMessageKey) payload).getPayloadMessageKey(); 078 if (payloadMessageKey != null) { 079 return payloadMessageKey; 080 } 081 } 082 return IMessage.super.getMessageKey(); 083 } 084 085 @Override 086 public int getRetryCount() { 087 return getHapiHeaders().getRetryCount(); 088 } 089 090 public T getPayloadWithRequestPartitionIdSetFromHeader(IDefaultPartitionSettings theDefaultPartitionSettings) { 091 RequestPartitionHeaderUtil.setRequestPartitionIdFromHeaderIfNotAlreadySet(this, theDefaultPartitionSettings); 092 return getPayload(); 093 } 094 095 public static <P> void addCustomHeaders(IMessage<P> theMessage, Map<String, ?> theCustomHeaders) { 096 if (theMessage instanceof BaseJsonMessage<P> baseJsonMessage) { 097 baseJsonMessage.getHapiHeaders().getCustomHeaders().putAll(theCustomHeaders); 098 } 099 } 100}