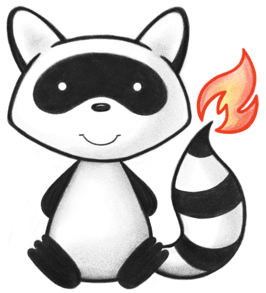
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.messaging.json; 021 022import ca.uhn.fhir.model.api.IModelJson; 023import com.fasterxml.jackson.annotation.JsonProperty; 024import org.springframework.messaging.MessageHeaders; 025 026import java.util.HashMap; 027import java.util.Map; 028 029import static java.util.Objects.isNull; 030 031/** 032 * This class is for holding headers for BaseJsonMessages. Any serializable data can be thrown into 033 * the header map. There are also three special headers, defined by the constants in this class, which are for use 034 * in message handling retrying. There are also matching helper functions for fetching those special variables; however 035 * they can also be accessed in standard map fashion with a `get` on the map. 036 */ 037public class HapiMessageHeaders implements IModelJson { 038 public static final String RETRY_COUNT_KEY = "retryCount"; 039 public static final String FIRST_FAILURE_KEY = "firstFailureTimestamp"; 040 public static final String LAST_FAILURE_KEY = "lastFailureTimestamp"; 041 042 @JsonProperty(RETRY_COUNT_KEY) 043 private Integer myRetryCount = 0; 044 045 @JsonProperty(FIRST_FAILURE_KEY) 046 private Long myFirstFailureTimestamp; 047 048 @JsonProperty(LAST_FAILURE_KEY) 049 private Long myLastFailureTimestamp; 050 051 @JsonProperty("customHeaders") 052 private final Map<String, Object> headers; 053 054 public HapiMessageHeaders(Map<String, Object> theHeaders) { 055 headers = theHeaders; 056 } 057 058 public HapiMessageHeaders() { 059 headers = new HashMap<>(); 060 } 061 062 public Integer getRetryCount() { 063 if (isNull(this.myRetryCount)) { 064 return 0; 065 } 066 return this.myRetryCount; 067 } 068 069 public Long getFirstFailureTimestamp() { 070 return this.myFirstFailureTimestamp; 071 } 072 073 public Long getLastFailureTimestamp() { 074 return this.myLastFailureTimestamp; 075 } 076 077 public void setRetryCount(Integer theRetryCount) { 078 this.myRetryCount = theRetryCount; 079 } 080 081 public void setLastFailureTimestamp(Long theLastFailureTimestamp) { 082 this.myLastFailureTimestamp = theLastFailureTimestamp; 083 } 084 085 public void setFirstFailureTimestamp(Long theFirstFailureTimestamp) { 086 this.myFirstFailureTimestamp = theFirstFailureTimestamp; 087 } 088 089 public Map<String, Object> getCustomHeaders() { 090 if (this.headers == null) { 091 return new HashMap<>(); 092 } 093 return this.headers; 094 } 095 096 public MessageHeaders toMessageHeaders() { 097 Map<String, Object> returnedHeaders = new HashMap<>(this.headers); 098 returnedHeaders.put(RETRY_COUNT_KEY, myRetryCount); 099 returnedHeaders.put(FIRST_FAILURE_KEY, myFirstFailureTimestamp); 100 returnedHeaders.put(LAST_FAILURE_KEY, myLastFailureTimestamp); 101 return new MessageHeaders(returnedHeaders); 102 } 103}