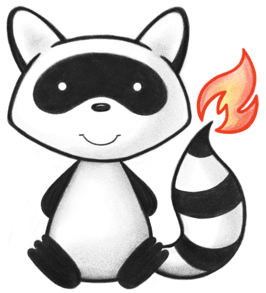
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.RuntimeResourceDefinition; 025import ca.uhn.fhir.i18n.Msg; 026import ca.uhn.fhir.rest.annotation.IdParam; 027import ca.uhn.fhir.rest.param.ParameterUtil; 028import ca.uhn.fhir.rest.server.IResourceProvider; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030 031import java.lang.reflect.Method; 032 033import static org.apache.commons.lang3.StringUtils.isNotBlank; 034 035public abstract class BaseOutcomeReturningMethodBindingWithResourceIdButNoResourceBody 036 extends BaseOutcomeReturningMethodBinding { 037 038 private String myResourceName; 039 private Integer myIdParameterIndex; 040 041 public BaseOutcomeReturningMethodBindingWithResourceIdButNoResourceBody( 042 Method theMethod, 043 FhirContext theContext, 044 Object theProvider, 045 Class<?> theMethodAnnotationType, 046 Class<? extends IBaseResource> theResourceTypeFromAnnotation, 047 String theResourceTypeNameFromAnnotation) { 048 super(theMethod, theContext, theMethodAnnotationType, theProvider); 049 050 Class<? extends IBaseResource> resourceType = theResourceTypeFromAnnotation; 051 if (resourceType != IBaseResource.class) { 052 RuntimeResourceDefinition def = theContext.getResourceDefinition(resourceType); 053 myResourceName = def.getName(); 054 } else if (isNotBlank(theResourceTypeNameFromAnnotation)) { 055 myResourceName = theResourceTypeNameFromAnnotation; 056 } else { 057 if (theProvider != null && theProvider instanceof IResourceProvider) { 058 RuntimeResourceDefinition def = 059 theContext.getResourceDefinition(((IResourceProvider) theProvider).getResourceType()); 060 myResourceName = def.getName(); 061 } else { 062 throw new ConfigurationException( 063 Msg.code(457) + "Can not determine resource type for method '" + theMethod.getName() 064 + "' on type " + theMethod.getDeclaringClass().getCanonicalName() 065 + " - Did you forget to include the resourceType() value on the @" 066 + theMethodAnnotationType.getSimpleName() + " method annotation?"); 067 } 068 } 069 070 myIdParameterIndex = ParameterUtil.findIdParameterIndex(theMethod, getContext()); 071 if (myIdParameterIndex == null) { 072 throw new ConfigurationException(Msg.code(458) + "Method '" + theMethod.getName() + "' on type '" 073 + theMethod.getDeclaringClass().getCanonicalName() + "' has no parameter annotated with the @" 074 + IdParam.class.getSimpleName() + " annotation"); 075 } 076 } 077 078 @Override 079 public String getResourceName() { 080 return myResourceName; 081 } 082 083 protected Integer getIdParameterIndex() { 084 return myIdParameterIndex; 085 } 086}