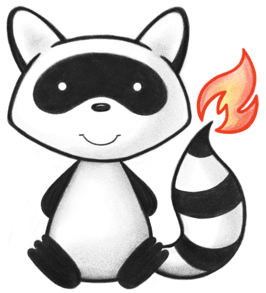
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.FhirVersionEnum; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.rest.annotation.Create; 026import ca.uhn.fhir.rest.api.RequestTypeEnum; 027import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 028import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 029import jakarta.annotation.Nonnull; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.hl7.fhir.instance.model.api.IIdType; 032 033import java.lang.reflect.Method; 034import java.util.Collections; 035import java.util.Set; 036 037import static org.apache.commons.lang3.StringUtils.isNotBlank; 038 039public class CreateMethodBinding extends BaseOutcomeReturningMethodBindingWithResourceParam { 040 041 public CreateMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 042 super(theMethod, theContext, Create.class, theProvider); 043 } 044 045 @Override 046 protected String getMatchingOperation() { 047 return null; 048 } 049 050 @Nonnull 051 @Override 052 public RestOperationTypeEnum getRestOperationType() { 053 return RestOperationTypeEnum.CREATE; 054 } 055 056 @Override 057 protected Set<RequestTypeEnum> provideAllowableRequestTypes() { 058 return Collections.singleton(RequestTypeEnum.POST); 059 } 060 061 @Override 062 protected void validateResourceIdAndUrlIdForNonConditionalOperation( 063 IBaseResource theResource, String theResourceId, String theUrlId, String theMatchUrl) { 064 if (isNotBlank(theUrlId)) { 065 String msg = getContext() 066 .getLocalizer() 067 .getMessage(BaseOutcomeReturningMethodBindingWithResourceParam.class, "idInUrlForCreate", theUrlId); 068 throw new InvalidRequestException(Msg.code(365) + msg); 069 } 070 if (getContext().getVersion().getVersion().isOlderThan(FhirVersionEnum.DSTU3)) { 071 if (isNotBlank(theResourceId)) { 072 String msg = getContext() 073 .getLocalizer() 074 .getMessage( 075 BaseOutcomeReturningMethodBindingWithResourceParam.class, 076 "idInBodyForCreate", 077 theResourceId); 078 throw new InvalidRequestException(Msg.code(366) + msg); 079 } 080 } else { 081 theResource.setId((IIdType) null); 082 } 083 } 084}