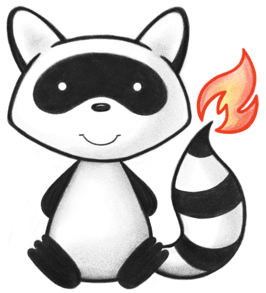
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.annotation.Delete; 024import ca.uhn.fhir.rest.api.RequestTypeEnum; 025import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 026import ca.uhn.fhir.rest.api.server.RequestDetails; 027import jakarta.annotation.Nonnull; 028 029import java.lang.reflect.Method; 030import java.util.Collections; 031import java.util.Set; 032 033public class DeleteMethodBinding extends BaseOutcomeReturningMethodBindingWithResourceIdButNoResourceBody { 034 035 public DeleteMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 036 super( 037 theMethod, 038 theContext, 039 theProvider, 040 Delete.class, 041 theMethod.getAnnotation(Delete.class).type(), 042 theMethod.getAnnotation(Delete.class).typeName()); 043 } 044 045 @Nonnull 046 @Override 047 public RestOperationTypeEnum getRestOperationType() { 048 return RestOperationTypeEnum.DELETE; 049 } 050 051 @Override 052 protected Set<RequestTypeEnum> provideAllowableRequestTypes() { 053 return Collections.singleton(RequestTypeEnum.DELETE); 054 } 055 056 @Override 057 protected boolean allowVoidReturnType() { 058 return true; 059 } 060 061 @Override 062 protected void addParametersForServerRequest(RequestDetails theRequest, Object[] theParams) { 063 theParams[getIdParameterIndex()] = theRequest.getId(); 064 } 065 066 @Override 067 protected String getMatchingOperation() { 068 return null; 069 } 070}