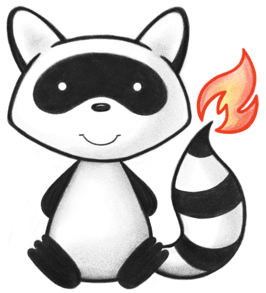
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.rest.annotation.RawParam; 023import ca.uhn.fhir.rest.api.server.RequestDetails; 024import ca.uhn.fhir.rest.param.QualifierDetails; 025import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 026import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 027import org.apache.commons.lang3.Validate; 028 029import java.lang.reflect.Method; 030import java.util.Arrays; 031import java.util.Collection; 032import java.util.HashMap; 033import java.util.List; 034import java.util.Map; 035 036public class RawParamsParameter implements IParameter { 037 038 private final List<IParameter> myAllMethodParameters; 039 040 public RawParamsParameter(List<IParameter> theParameters) { 041 myAllMethodParameters = theParameters; 042 } 043 044 @Override 045 public Object translateQueryParametersIntoServerArgument( 046 RequestDetails theRequest, BaseMethodBinding theMethodBinding) 047 throws InternalErrorException, InvalidRequestException { 048 HashMap<String, List<String>> retVal = null; 049 050 for (String nextName : theRequest.getParameters().keySet()) { 051 if (nextName.startsWith("_")) { 052 continue; 053 } 054 055 QualifierDetails qualifiers = QualifierDetails.extractQualifiersFromParameterName(nextName); 056 057 boolean alreadyCaptured = false; 058 for (IParameter nextParameter : myAllMethodParameters) { 059 if (nextParameter instanceof SearchParameter) { 060 SearchParameter nextSearchParam = (SearchParameter) nextParameter; 061 if (nextSearchParam.getName().equals(qualifiers.getParamName())) { 062 if (qualifiers.passes( 063 nextSearchParam.getQualifierWhitelist(), nextSearchParam.getQualifierBlacklist())) { 064 alreadyCaptured = true; 065 break; 066 } 067 } 068 } 069 } 070 071 if (!alreadyCaptured) { 072 if (retVal == null) { 073 retVal = new HashMap<>(); 074 } 075 retVal.put(nextName, Arrays.asList(theRequest.getParameters().get(nextName))); 076 } 077 } 078 079 return retVal; 080 } 081 082 @Override 083 public void initializeTypes( 084 Method theMethod, 085 Class<? extends Collection<?>> theOuterCollectionType, 086 Class<? extends Collection<?>> theInnerCollectionType, 087 Class<?> theParameterType) { 088 Validate.isTrue( 089 theParameterType.equals(Map.class), 090 "Parameter with @" + RawParam.class + " must be of type Map<String, List<String>>"); 091 } 092}