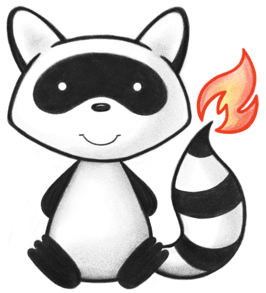
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.model.base.resource.BaseOperationOutcome; 026import ca.uhn.fhir.model.valueset.BundleTypeEnum; 027import ca.uhn.fhir.rest.annotation.Transaction; 028import ca.uhn.fhir.rest.annotation.TransactionParam; 029import ca.uhn.fhir.rest.api.RequestTypeEnum; 030import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 031import ca.uhn.fhir.rest.api.server.IBundleProvider; 032import ca.uhn.fhir.rest.api.server.IRestfulServer; 033import ca.uhn.fhir.rest.api.server.RequestDetails; 034import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 035import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 036import ca.uhn.fhir.rest.server.method.TransactionParameter.ParamStyle; 037import jakarta.annotation.Nonnull; 038import org.hl7.fhir.instance.model.api.IBaseResource; 039 040import java.lang.reflect.Method; 041import java.util.List; 042 043import static org.apache.commons.lang3.StringUtils.isNotBlank; 044 045public class TransactionMethodBinding extends BaseResourceReturningMethodBinding { 046 047 private int myTransactionParamIndex; 048 private ParamStyle myTransactionParamStyle; 049 050 public TransactionMethodBinding(Method theMethod, FhirContext theContext, Object theProvider) { 051 super(null, theMethod, theContext, theProvider); 052 053 myTransactionParamIndex = -1; 054 int index = 0; 055 for (IParameter next : getParameters()) { 056 if (next instanceof TransactionParameter) { 057 if (myTransactionParamIndex != -1) { 058 throw new ConfigurationException(Msg.code(372) + "Method '" + theMethod.getName() + "' in type " 059 + theMethod.getDeclaringClass().getCanonicalName() 060 + " has multiple parameters annotated with the @" 061 + TransactionParam.class + " annotation, exactly one is required for @" + Transaction.class 062 + " methods"); 063 } 064 myTransactionParamIndex = index; 065 myTransactionParamStyle = ((TransactionParameter) next).getParamStyle(); 066 } 067 index++; 068 } 069 070 if (myTransactionParamIndex == -1) { 071 throw new ConfigurationException(Msg.code(373) + "Method '" + theMethod.getName() + "' in type " 072 + theMethod.getDeclaringClass().getCanonicalName() 073 + " does not have a parameter annotated with the @" + TransactionParam.class + " annotation"); 074 } 075 } 076 077 @Nonnull 078 @Override 079 public RestOperationTypeEnum getRestOperationType() { 080 return RestOperationTypeEnum.TRANSACTION; 081 } 082 083 @Override 084 protected BundleTypeEnum getResponseBundleType() { 085 return BundleTypeEnum.TRANSACTION_RESPONSE; 086 } 087 088 @Override 089 public ReturnTypeEnum getReturnType() { 090 return ReturnTypeEnum.BUNDLE; 091 } 092 093 @Override 094 public MethodMatchEnum incomingServerRequestMatchesMethod(RequestDetails theRequest) { 095 if (theRequest.getRequestType() != RequestTypeEnum.POST) { 096 return MethodMatchEnum.NONE; 097 } 098 if (isNotBlank(theRequest.getOperation())) { 099 return MethodMatchEnum.NONE; 100 } 101 if (isNotBlank(theRequest.getResourceName())) { 102 return MethodMatchEnum.NONE; 103 } 104 return MethodMatchEnum.EXACT; 105 } 106 107 @SuppressWarnings("unchecked") 108 @Override 109 public Object invokeServer(IRestfulServer<?> theServer, RequestDetails theRequest, Object[] theMethodParams) 110 throws InvalidRequestException, InternalErrorException { 111 112 /* 113 * The design of HAPI's transaction method for DSTU1 support assumed that a transaction was just an update on a 114 * bunch of resources (because that's what it was), but in DSTU2 transaction has become much more broad, so we 115 * no longer hold the user's hand much here. 116 */ 117 if (myTransactionParamStyle == ParamStyle.RESOURCE_BUNDLE) { 118 // This is the DSTU2 style 119 Object response = invokeServerMethod(theRequest, theMethodParams); 120 return response; 121 } 122 123 // Call the server implementation method 124 Object response = invokeServerMethod(theRequest, theMethodParams); 125 IBundleProvider retVal = toResourceList(response); 126 127 /* 128 * int offset = 0; if (retVal.size() != resources.size()) { if (retVal.size() > 0 && retVal.getResources(0, 129 * 1).get(0) instanceof OperationOutcome) { offset = 1; } else { throw new 130 * InternalErrorException("Transaction bundle contained " + resources.size() + 131 * " entries, but server method response contained " + retVal.size() + " entries (must be the same)"); } } 132 */ 133 134 List<IBaseResource> retResources = retVal.getAllResources(); 135 for (int i = 0; i < retResources.size(); i++) { 136 IBaseResource newRes = retResources.get(i); 137 if (newRes.getIdElement() == null || newRes.getIdElement().isEmpty()) { 138 if (!(newRes instanceof BaseOperationOutcome)) { 139 throw new InternalErrorException(Msg.code(374) + "Transaction method returned resource at index " 140 + i + " with no id specified - IResource#setId(IdDt)"); 141 } 142 } 143 } 144 145 return retVal; 146 } 147 148 @Override 149 protected void populateRequestDetailsForInterceptor(RequestDetails theRequestDetails, Object[] theMethodParams) { 150 super.populateRequestDetailsForInterceptor(theRequestDetails, theMethodParams); 151 152 /* 153 * If the method has no parsed resource parameter, we parse here in order to have something for the interceptor. 154 */ 155 IBaseResource resource; 156 if (myTransactionParamIndex != -1) { 157 resource = (IBaseResource) theMethodParams[myTransactionParamIndex]; 158 } else { 159 Class<? extends IBaseResource> resourceType = 160 getContext().getResourceDefinition("Bundle").getImplementingClass(); 161 resource = ResourceParameter.parseResourceFromRequest(theRequestDetails, this, resourceType); 162 } 163 164 theRequestDetails.setResource(resource); 165 } 166}