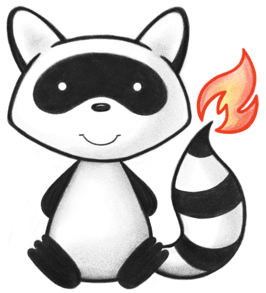
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.RuntimeResourceDefinition; 025import ca.uhn.fhir.i18n.Msg; 026import ca.uhn.fhir.model.api.IResource; 027import ca.uhn.fhir.rest.annotation.TransactionParam; 028import ca.uhn.fhir.rest.api.server.RequestDetails; 029import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 030import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 031import ca.uhn.fhir.util.BundleUtil; 032import org.hl7.fhir.instance.model.api.IBaseBundle; 033import org.hl7.fhir.instance.model.api.IBaseResource; 034 035import java.lang.reflect.Method; 036import java.lang.reflect.Modifier; 037import java.util.Collection; 038import java.util.List; 039 040public class TransactionParameter implements IParameter { 041 042 // private static final org.slf4j.Logger ourLog = org.slf4j.LoggerFactory.getLogger(TransactionParameter.class); 043 private FhirContext myContext; 044 private ParamStyle myParamStyle; 045 private Class<? extends IBaseResource> myResourceBundleType; 046 047 TransactionParameter(FhirContext theContext) { 048 myContext = theContext; 049 } 050 051 private String createParameterTypeError(Method theMethod) { 052 return "Method '" + theMethod.getName() + "' in type '" 053 + theMethod.getDeclaringClass().getCanonicalName() + "' is annotated with @" 054 + TransactionParam.class.getName() + " but is not of type Bundle, IBaseResource, IBaseBundle, or List<" 055 + IResource.class.getCanonicalName() + ">"; 056 } 057 058 @Override 059 public void initializeTypes( 060 Method theMethod, 061 Class<? extends Collection<?>> theOuterCollectionType, 062 Class<? extends Collection<?>> theInnerCollectionType, 063 Class<?> theParameterType) { 064 if (theOuterCollectionType != null) { 065 throw new ConfigurationException(Msg.code(429) + "Method '" + theMethod.getName() + "' in type '" 066 + theMethod.getDeclaringClass().getCanonicalName() + "' is annotated with @" 067 + TransactionParam.class.getName() + " but can not be a collection of collections"); 068 } 069 if (Modifier.isInterface(theParameterType.getModifiers()) == false 070 && IBaseResource.class.isAssignableFrom(theParameterType)) { 071 @SuppressWarnings("unchecked") 072 Class<? extends IBaseResource> parameterType = (Class<? extends IBaseResource>) theParameterType; 073 RuntimeResourceDefinition def = myContext.getResourceDefinition(parameterType); 074 if ("Bundle".equals(def.getName())) { 075 myParamStyle = ParamStyle.RESOURCE_BUNDLE; 076 myResourceBundleType = parameterType; 077 return; 078 } 079 } else if (theParameterType.equals(IBaseResource.class) || theParameterType.equals(IBaseBundle.class)) { 080 myParamStyle = ParamStyle.RESOURCE_BUNDLE; 081 myResourceBundleType = myContext.getResourceDefinition("Bundle").getImplementingClass(); 082 return; 083 } else if (theInnerCollectionType != null) { 084 if (theInnerCollectionType.equals(List.class) == false) { 085 throw new ConfigurationException(Msg.code(430) + createParameterTypeError(theMethod)); 086 } 087 if (theParameterType.equals(IResource.class) == false) { 088 throw new ConfigurationException(Msg.code(431) + createParameterTypeError(theMethod)); 089 } 090 myParamStyle = ParamStyle.RESOURCE_LIST; 091 return; 092 } 093 094 throw new ConfigurationException(Msg.code(432) + createParameterTypeError(theMethod)); 095 } 096 097 @Override 098 public Object translateQueryParametersIntoServerArgument( 099 RequestDetails theRequest, BaseMethodBinding theMethodBinding) 100 throws InternalErrorException, InvalidRequestException { 101 IBaseResource parsedBundle = 102 ResourceParameter.parseResourceFromRequest(theRequest, theMethodBinding, myResourceBundleType); 103 104 switch (myParamStyle) { 105 case RESOURCE_LIST: 106 return BundleUtil.toListOfResources(myContext, (IBaseBundle) parsedBundle); 107 case RESOURCE_BUNDLE: 108 default: 109 assert myParamStyle == ParamStyle.RESOURCE_BUNDLE; 110 break; 111 } 112 113 return parsedBundle; 114 } 115 116 ParamStyle getParamStyle() { 117 return myParamStyle; 118 } 119 120 public enum ParamStyle { 121 /** 122 * New style bundle (defined in hapi-fhir-structures-* as a resource definition itself 123 */ 124 RESOURCE_BUNDLE, 125 /** 126 * List of resources 127 */ 128 RESOURCE_LIST 129 } 130}