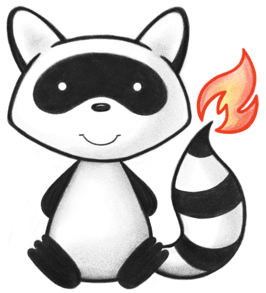
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.method; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.valueset.BundleTypeEnum; 024import ca.uhn.fhir.rest.annotation.OperationParam; 025import ca.uhn.fhir.rest.annotation.Validate; 026import ca.uhn.fhir.rest.api.Constants; 027import ca.uhn.fhir.rest.api.EncodingEnum; 028import ca.uhn.fhir.util.ParametersUtil; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030 031import java.lang.annotation.Annotation; 032import java.lang.reflect.Method; 033import java.util.ArrayList; 034import java.util.List; 035 036public class ValidateMethodBindingDstu2Plus extends OperationMethodBinding { 037 038 public ValidateMethodBindingDstu2Plus( 039 Class<?> theReturnResourceType, 040 Class<? extends IBaseResource> theReturnTypeFromRp, 041 Method theMethod, 042 FhirContext theContext, 043 Object theProvider, 044 Validate theAnnotation) { 045 super( 046 theReturnResourceType, 047 theReturnTypeFromRp, 048 theMethod, 049 theContext, 050 theProvider, 051 true, 052 false, 053 Constants.EXTOP_VALIDATE, 054 theAnnotation.type(), 055 null, 056 new OperationParam[0], 057 BundleTypeEnum.COLLECTION, 058 false); 059 060 List<IParameter> newParams = new ArrayList<>(); 061 int idx = 0; 062 for (IParameter next : getParameters()) { 063 if (next instanceof ResourceParameter) { 064 if (IBaseResource.class.isAssignableFrom(((ResourceParameter) next).getResourceType())) { 065 Class<?> parameterType = theMethod.getParameterTypes()[idx]; 066 if (String.class.equals(parameterType) || EncodingEnum.class.equals(parameterType)) { 067 newParams.add(next); 068 } else { 069 Annotation[] parameterAnnotations = theMethod.getParameterAnnotations()[idx]; 070 String description = ParametersUtil.extractDescription(parameterAnnotations); 071 List<String> examples = ParametersUtil.extractExamples(parameterAnnotations); 072 OperationParameter parameter = new OperationParameter( 073 theContext, 074 Constants.EXTOP_VALIDATE, 075 Constants.EXTOP_VALIDATE_RESOURCE, 076 0, 077 1, 078 description, 079 examples); 080 parameter.initializeTypes(theMethod, null, null, parameterType); 081 newParams.add(parameter); 082 } 083 } else { 084 newParams.add(next); 085 } 086 } else { 087 newParams.add(next); 088 } 089 idx++; 090 } 091 setParameters(newParams); 092 } 093}