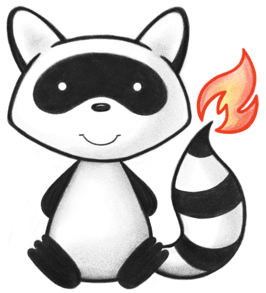
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.provider; 021 022import ca.uhn.fhir.rest.annotation.Operation; 023import ca.uhn.fhir.rest.annotation.OperationParam; 024import ca.uhn.fhir.rest.api.Constants; 025import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 026import org.hl7.fhir.instance.model.api.IBaseBundle; 027import org.hl7.fhir.instance.model.api.IBaseCoding; 028import org.hl7.fhir.instance.model.api.IBaseReference; 029import org.hl7.fhir.instance.model.api.IPrimitiveType; 030 031import java.util.List; 032 033/** 034 * This class implements the Observation 035 * <a href="http://hl7.org/fhir/observation-operation-lastn.html">$lastn</a> operation. 036 * <p> 037 * It is does not implement the actual storage logic for this operation, but can be 038 * subclassed to provide this functionality. 039 * </p> 040 * 041 * @since 4.1.0 042 */ 043public abstract class BaseLastNProvider { 044 045 @Operation(name = Constants.OPERATION_LASTN, typeName = "Observation", idempotent = true) 046 public IBaseBundle lastN( 047 ServletRequestDetails theRequestDetails, 048 @OperationParam(name = "subject", typeName = "reference", min = 0, max = 1) IBaseReference theSubject, 049 @OperationParam(name = "category", typeName = "coding", min = 0, max = OperationParam.MAX_UNLIMITED) 050 List<IBaseCoding> theCategories, 051 @OperationParam(name = "code", typeName = "coding", min = 0, max = OperationParam.MAX_UNLIMITED) 052 List<IBaseCoding> theCodes, 053 @OperationParam(name = "max", typeName = "integer", min = 0, max = 1) IPrimitiveType<Integer> theMax) { 054 return processLastN(theSubject, theCategories, theCodes, theMax); 055 } 056 057 /** 058 * Subclasses should implement this method 059 */ 060 protected abstract IBaseBundle processLastN( 061 IBaseReference theSubject, 062 List<IBaseCoding> theCategories, 063 List<IBaseCoding> theCodes, 064 IPrimitiveType<Integer> theMax); 065}