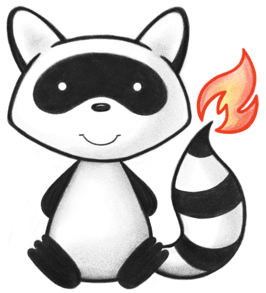
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.provider; 021 022public class ProviderConstants { 023 public static final String SUBSCRIPTION_TRIGGERING_PARAM_RESOURCE_ID = "resourceId"; 024 public static final String SUBSCRIPTION_TRIGGERING_PARAM_SEARCH_URL = "searchUrl"; 025 026 /** 027 * Operation name: add partition 028 */ 029 public static final String PARTITION_MANAGEMENT_CREATE_PARTITION = "$partition-management-create-partition"; 030 031 /** 032 * Operation name: update partition 033 */ 034 public static final String PARTITION_MANAGEMENT_UPDATE_PARTITION = "$partition-management-update-partition"; 035 036 /** 037 * Operation name: update partition 038 */ 039 public static final String PARTITION_MANAGEMENT_DELETE_PARTITION = "$partition-management-delete-partition"; 040 041 /** 042 * Operation name: read partition 043 */ 044 public static final String PARTITION_MANAGEMENT_READ_PARTITION = "$partition-management-read-partition"; 045 046 /** 047 * Operation name: list partitions 048 */ 049 public static final String PARTITION_MANAGEMENT_LIST_PARTITIONS = "$partition-management-list-partitions"; 050 051 public static final String PARTITION_MANAGEMENT_PARTITION_ID = "id"; 052 public static final String PARTITION_MANAGEMENT_PARTITION_NAME = "name"; 053 public static final String PARTITION_MANAGEMENT_PARTITION_DESC = "description"; 054 055 public static final String DEFAULT_PARTITION_NAME = "DEFAULT"; 056 public static final String ALL_PARTITIONS_TENANT_NAME = "_ALL"; 057 058 /** 059 * Operation name: diff 060 */ 061 public static final String DIFF_OPERATION_NAME = "$diff"; 062 063 public static final String DIFF_FROM_VERSION_PARAMETER = "fromVersion"; 064 065 public static final String DIFF_FROM_PARAMETER = "from"; 066 public static final String DIFF_TO_PARAMETER = "to"; 067 public static final String DIFF_INCLUDE_META_PARAMETER = "includeMeta"; 068 069 /** 070 * MDM Operations 071 */ 072 public static final String PATIENT_MATCH = "$match"; 073 074 public static final String MDM_MATCH = "$mdm-match"; 075 public static final String MDM_MATCH_RESOURCE = "resource"; 076 public static final String MDM_RESOURCE_TYPE = "resourceType"; 077 public static final String MDM_MERGE_GOLDEN_RESOURCES = "$mdm-merge-golden-resources"; 078 public static final String MDM_MERGE_GR_FROM_GOLDEN_RESOURCE_ID = "fromGoldenResourceId"; 079 public static final String MDM_MERGE_GR_TO_GOLDEN_RESOURCE_ID = "toGoldenResourceId"; 080 public static final String MDM_MERGE_RESOURCE = "resource"; 081 082 public static final String MDM_UPDATE_LINK = "$mdm-update-link"; 083 public static final String MDM_UPDATE_LINK_GOLDEN_RESOURCE_ID = "goldenResourceId"; 084 public static final String MDM_UPDATE_LINK_RESOURCE_ID = "resourceId"; 085 public static final String MDM_UPDATE_LINK_MATCH_RESULT = "matchResult"; 086 087 public static final String MDM_CREATE_LINK = "$mdm-create-link"; 088 public static final String MDM_CREATE_LINK_GOLDEN_RESOURCE_ID = "goldenResourceId"; 089 public static final String MDM_CREATE_LINK_RESOURCE_ID = "resourceId"; 090 public static final String MDM_CREATE_LINK_MATCH_RESULT = "matchResult"; 091 092 public static final String MDM_QUERY_LINKS = "$mdm-query-links"; 093 public static final String MDM_LINK_HISTORY = "$mdm-link-history"; 094 public static final String MDM_QUERY_LINKS_GOLDEN_RESOURCE_ID = "goldenResourceId"; 095 public static final String MDM_QUERY_LINKS_RESOURCE_ID = "resourceId"; 096 public static final String MDM_QUERY_PARTITION_IDS = "partitionIds"; 097 public static final String MDM_QUERY_LINKS_MATCH_RESULT = "matchResult"; 098 public static final String MDM_QUERY_LINKS_LINK_SOURCE = "linkSource"; 099 100 public static final String MDM_DUPLICATE_GOLDEN_RESOURCES = "$mdm-duplicate-golden-resources"; 101 public static final String MDM_NOT_DUPLICATE = "$mdm-not-duplicate"; 102 103 public static final String OPERATION_MDM_CLEAR = "$mdm-clear"; 104 public static final String OPERATION_MDM_CLEAR_RESOURCE_NAME = "resourceType"; 105 public static final String OPERATION_MDM_BATCH_SIZE = "batchSize"; 106 public static final String OPERATION_MDM_SUBMIT = "$mdm-submit"; 107 public static final String OPERATION_MDM_SUBMIT_OUT_PARAM_SUBMITTED = "submitted"; 108 public static final String MDM_BATCH_RUN_CRITERIA = "criteria"; 109 public static final String MDM_BATCH_RUN_RESOURCE_TYPE = "resourceType"; 110 111 /** 112 * Clinical Reasoning Operations 113 */ 114 public static final String CR_OPERATION_EVALUATE_MEASURE = "$evaluate-measure"; 115 116 public static final String CR_OPERATION_CARE_GAPS = "$care-gaps"; 117 public static final String CR_OPERATION_SUBMIT_DATA = "$submit-data"; 118 public static final String CR_OPERATION_EVALUATE = "$evaluate"; 119 public static final String CR_OPERATION_CQL = "$cql"; 120 public static final String CR_OPERATION_APPLY = "$apply"; 121 public static final String CR_OPERATION_R5_APPLY = "$r5.apply"; 122 public static final String CR_OPERATION_PREPOPULATE = "$prepopulate"; 123 public static final String CR_OPERATION_POPULATE = "$populate"; 124 public static final String CR_OPERATION_EXTRACT = "$extract"; 125 public static final String CR_OPERATION_PACKAGE = "$package"; 126 public static final String CR_OPERATION_QUESTIONNAIRE = "$questionnaire"; 127 public static final String CR_OPERATION_COLLECTDATA = "$collect-data"; 128 public static final String CR_OPERATION_DATAREQUIREMENTS = "$data-requirements"; 129 /** 130 * Operation name for the $meta operation 131 */ 132 public static final String OPERATION_META = "$meta"; 133 134 /** 135 * Operation name for the $expunge operation 136 */ 137 public static final String OPERATION_EXPUNGE = "$expunge"; 138 139 /** 140 * Parameter name for the $expunge operation 141 */ 142 public static final String OPERATION_EXPUNGE_PARAM_LIMIT = "limit"; 143 /** 144 * Parameter name for the $expunge operation 145 */ 146 public static final String OPERATION_EXPUNGE_PARAM_EXPUNGE_DELETED_RESOURCES = "expungeDeletedResources"; 147 /** 148 * Parameter name for the $expunge operation 149 */ 150 public static final String OPERATION_EXPUNGE_PARAM_EXPUNGE_PREVIOUS_VERSIONS = "expungePreviousVersions"; 151 /** 152 * Parameter name for the $expunge operation 153 */ 154 public static final String OPERATION_EXPUNGE_PARAM_EXPUNGE_EVERYTHING = "expungeEverything"; 155 /** 156 * Output parameter name for the $expunge operation 157 */ 158 public static final String OPERATION_EXPUNGE_OUT_PARAM_EXPUNGE_COUNT = "count"; 159 160 /** 161 * Operation name for the $delete-expunge operation 162 */ 163 public static final String OPERATION_DELETE_EXPUNGE = "$delete-expunge"; 164 165 /** 166 * url of resources to delete for the $delete-expunge operation 167 */ 168 public static final String OPERATION_DELETE_EXPUNGE_URL = "url"; 169 170 /** 171 * Number of resources to delete at a time for the $delete-expunge operation 172 */ 173 public static final String OPERATION_DELETE_BATCH_SIZE = "batchSize"; 174 /** 175 * Should we cascade the $delete-expunge operation 176 */ 177 public static final String OPERATION_DELETE_CASCADE = "cascade"; 178 /** 179 * How many rounds for the $delete-expunge operation 180 */ 181 public static final String OPERATION_DELETE_CASCADE_MAX_ROUNDS = "cascadeMaxRounds"; 182 183 /** 184 * The Spring Batch job id of the delete expunge job created by a $delete-expunge operation 185 */ 186 public static final String OPERATION_BATCH_RESPONSE_JOB_ID = "jobId"; 187 188 /** 189 * Operation name for the $reindex operation 190 */ 191 public static final String OPERATION_REINDEX = "$reindex"; 192 193 /** 194 * Operation name for the $reindex operation 195 */ 196 public static final String OPERATION_REINDEX_DRYRUN = "$reindex-dryrun"; 197 198 /** 199 * Operation name for the $invalidate-expansion operation 200 */ 201 public static final String OPERATION_INVALIDATE_EXPANSION = "$invalidate-expansion"; 202 203 /** 204 * url of resources to delete for the $delete-expunge operation 205 */ 206 public static final String OPERATION_REINDEX_PARAM_URL = "url"; 207 208 /** 209 * Number of resources to delete at a time for the $delete-expunge operation 210 */ 211 public static final String OPERATION_REINDEX_PARAM_BATCH_SIZE = "batchSize"; 212 213 /** 214 * Whether all resource types should be reindexed 215 */ 216 @Deprecated(since = "7.3.4") 217 public static final String OPERATION_REINDEX_PARAM_EVERYTHING = "everything"; 218 219 /** 220 * The Spring Batch job id of the delete expunge job created by a $delete-expunge operation 221 */ 222 public static final String OPERATION_REINDEX_RESPONSE_JOB_ID = "jobId"; 223 224 /** 225 * Operation name for the $reindex-terminology operation 226 */ 227 public static final String OPERATION_REINDEX_TERMINOLOGY = "$reindex-terminology"; 228 229 @Deprecated 230 public static final String MARK_ALL_RESOURCES_FOR_REINDEXING = "$mark-all-resources-for-reindexing"; 231 /** 232 * @see ProviderConstants#OPERATION_REINDEX 233 * @deprecated 234 */ 235 @Deprecated 236 public static final String PERFORM_REINDEXING_PASS = "$perform-reindexing-pass"; 237 238 /** 239 * Operation name for the "$export-poll-status" operation 240 */ 241 public static final String OPERATION_EXPORT_POLL_STATUS = "$export-poll-status"; 242 243 /** 244 * Operation name for the "$export" operation 245 */ 246 public static final String OPERATION_EXPORT = "$export"; 247 248 /** 249 * Operation name for the "$hapi.fhir.replace-references" operation 250 */ 251 public static final String OPERATION_REPLACE_REFERENCES = "$hapi.fhir.replace-references"; 252 253 /** 254 * Parameter for source reference of the "$hapi.fhir.replace-references" operation 255 */ 256 public static final String OPERATION_REPLACE_REFERENCES_PARAM_SOURCE_REFERENCE_ID = "source-reference-id"; 257 258 /** 259 * Parameter for target reference of the "$hapi.fhir.replace-references" operation 260 */ 261 public static final String OPERATION_REPLACE_REFERENCES_PARAM_TARGET_REFERENCE_ID = "target-reference-id"; 262 263 /** 264 * If the request is being performed synchronously and the number of resources that need to change 265 * exceeds this amount, the operation will fail with 412 Precondition Failed. 266 */ 267 public static final String OPERATION_REPLACE_REFERENCES_RESOURCE_LIMIT = "resource-limit"; 268 269 /** 270 * $hapi.fhir.replace-references output Parameters names 271 */ 272 public static final String OPERATION_REPLACE_REFERENCES_OUTPUT_PARAM_TASK = "task"; 273 274 public static final String OPERATION_REPLACE_REFERENCES_OUTPUT_PARAM_OUTCOME = "outcome"; 275 276 /** 277 * Operation name for the Resource "$merge" operation 278 * Hapi-fhir use is based on https://www.hl7.org/fhir/patient-operation-merge.html 279 */ 280 public static final String OPERATION_MERGE = "$merge"; 281 /** 282 * Patient $merge operation parameters 283 */ 284 public static final String OPERATION_MERGE_PARAM_SOURCE_PATIENT = "source-patient"; 285 286 public static final String OPERATION_MERGE_PARAM_SOURCE_PATIENT_IDENTIFIER = "source-patient-identifier"; 287 public static final String OPERATION_MERGE_PARAM_TARGET_PATIENT = "target-patient"; 288 public static final String OPERATION_MERGE_PARAM_TARGET_PATIENT_IDENTIFIER = "target-patient-identifier"; 289 public static final String OPERATION_MERGE_PARAM_RESULT_PATIENT = "result-patient"; 290 public static final String OPERATION_MERGE_PARAM_BATCH_SIZE = "batch-size"; 291 public static final String OPERATION_MERGE_PARAM_PREVIEW = "preview"; 292 public static final String OPERATION_MERGE_PARAM_DELETE_SOURCE = "delete-source"; 293 public static final String OPERATION_MERGE_OUTPUT_PARAM_INPUT = "input"; 294 public static final String OPERATION_MERGE_OUTPUT_PARAM_OUTCOME = OPERATION_REPLACE_REFERENCES_OUTPUT_PARAM_OUTCOME; 295 public static final String OPERATION_MERGE_OUTPUT_PARAM_RESULT = "result"; 296 public static final String OPERATION_MERGE_OUTPUT_PARAM_TASK = OPERATION_REPLACE_REFERENCES_OUTPUT_PARAM_TASK; 297 298 public static final String HAPI_BATCH_JOB_ID_SYSTEM = "http://hapifhir.io/batch/jobId"; 299 public static final String OPERATION_REPLACE_REFERENCES_RESOURCE_LIMIT_DEFAULT_STRING = "512"; 300 public static final Integer OPERATION_REPLACE_REFERENCES_RESOURCE_LIMIT_DEFAULT = 301 Integer.parseInt(OPERATION_REPLACE_REFERENCES_RESOURCE_LIMIT_DEFAULT_STRING); 302}