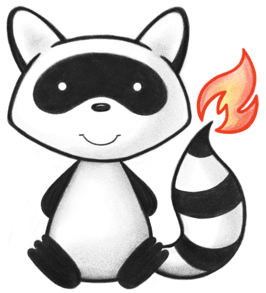
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.util; 021 022import ca.uhn.fhir.context.ComboSearchParamType; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.RuntimeResourceDefinition; 025import ca.uhn.fhir.context.RuntimeSearchParam; 026import ca.uhn.fhir.context.phonetic.IPhoneticEncoder; 027import ca.uhn.fhir.i18n.Msg; 028import jakarta.annotation.Nonnull; 029import jakarta.annotation.Nullable; 030import org.apache.commons.lang3.Validate; 031import org.hl7.fhir.instance.model.api.IIdType; 032 033import java.util.ArrayList; 034import java.util.List; 035import java.util.Optional; 036import java.util.Set; 037 038import static ca.uhn.fhir.rest.server.util.ISearchParamRegistry.isAllowedForContext; 039 040public class FhirContextSearchParamRegistry implements ISearchParamRegistry { 041 042 private final List<RuntimeSearchParam> myExtraSearchParams = new ArrayList<>(); 043 private final FhirContext myCtx; 044 045 /** 046 * Constructor 047 */ 048 public FhirContextSearchParamRegistry(@Nonnull FhirContext theCtx) { 049 Validate.notNull(theCtx, "theCtx must not be null"); 050 myCtx = theCtx; 051 } 052 053 @Override 054 public void forceRefresh() { 055 // nothing 056 } 057 058 @Override 059 public RuntimeSearchParam getActiveSearchParam( 060 @Nonnull String theResourceName, 061 @Nonnull String theParamName, 062 @Nonnull SearchParamLookupContextEnum theContext) { 063 return getActiveSearchParams(theResourceName, theContext).get(theParamName); 064 } 065 066 @Override 067 public ResourceSearchParams getActiveSearchParams( 068 @Nonnull String theResourceName, @Nonnull SearchParamLookupContextEnum theContext) { 069 ResourceSearchParams retval = new ResourceSearchParams(theResourceName); 070 RuntimeResourceDefinition nextResDef = myCtx.getResourceDefinition(theResourceName); 071 for (RuntimeSearchParam nextSp : nextResDef.getSearchParams()) { 072 if (isAllowedForContext(nextSp, theContext)) { 073 retval.put(nextSp.getName(), nextSp); 074 } 075 } 076 077 for (RuntimeSearchParam next : myExtraSearchParams) { 078 if (isAllowedForContext(next, theContext)) { 079 retval.put(next.getName(), next); 080 } 081 } 082 083 return retval; 084 } 085 086 public void addSearchParam(RuntimeSearchParam theSearchParam) { 087 myExtraSearchParams.add(theSearchParam); 088 } 089 090 @Override 091 public List<RuntimeSearchParam> getActiveComboSearchParams( 092 @Nonnull String theResourceName, 093 @Nonnull Set<String> theParamNames, 094 @Nonnull SearchParamLookupContextEnum theContext) { 095 throw new UnsupportedOperationException(Msg.code(2066)); 096 } 097 098 @Nullable 099 @Override 100 public RuntimeSearchParam getActiveSearchParamByUrl( 101 @Nonnull String theUrl, @Nonnull SearchParamLookupContextEnum theContext) { 102 // simple implementation for test support 103 return myCtx.getResourceTypes().stream() 104 .flatMap(type -> getActiveSearchParams(type, theContext).values().stream()) 105 .filter(rsp -> theUrl.equals(rsp.getUri())) 106 .findFirst() 107 .orElse(null); 108 } 109 110 @Override 111 public List<RuntimeSearchParam> getActiveComboSearchParams( 112 @Nonnull String theResourceName, @Nonnull SearchParamLookupContextEnum theContext) { 113 throw new UnsupportedOperationException(Msg.code(2068)); 114 } 115 116 @Override 117 public List<RuntimeSearchParam> getActiveComboSearchParams( 118 @Nonnull String theResourceName, 119 @Nonnull ComboSearchParamType theParamType, 120 @Nonnull SearchParamLookupContextEnum theContext) { 121 throw new UnsupportedOperationException(Msg.code(2209)); 122 } 123 124 @Override 125 public Optional<RuntimeSearchParam> getActiveComboSearchParamById( 126 @Nonnull String theResourceName, @Nonnull IIdType theId) { 127 throw new UnsupportedOperationException(Msg.code(2211)); 128 } 129 130 @Override 131 public void setPhoneticEncoder(IPhoneticEncoder thePhoneticEncoder) { 132 // nothing 133 } 134}