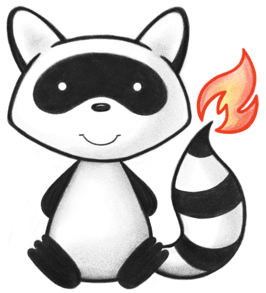
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.util; 021 022import ca.uhn.fhir.rest.api.RequestTypeEnum; 023import ca.uhn.fhir.rest.server.servlet.ServletRequestDetails; 024import ca.uhn.fhir.rest.server.servlet.ServletSubRequestDetails; 025import com.google.common.collect.ArrayListMultimap; 026import org.apache.http.NameValuePair; 027 028import java.util.Collection; 029import java.util.HashMap; 030import java.util.List; 031import java.util.Map; 032 033public class ServletRequestUtil { 034 public static ServletSubRequestDetails getServletSubRequestDetails( 035 ServletRequestDetails theRequestDetails, 036 String url, 037 String theVerb, 038 ArrayListMultimap<String, String> theParamValues) { 039 ServletSubRequestDetails requestDetails = new ServletSubRequestDetails(theRequestDetails); 040 requestDetails.setServletRequest(theRequestDetails.getServletRequest()); 041 requestDetails.setRequestType(RequestTypeEnum.valueOf(theVerb)); 042 requestDetails.setServer(theRequestDetails.getServer()); 043 requestDetails.setRestOperationType(theRequestDetails.getRestOperationType()); 044 045 int qIndex = url.indexOf('?'); 046 requestDetails.setParameters(new HashMap<>()); 047 if (qIndex != -1) { 048 String params = url.substring(qIndex); 049 List<NameValuePair> parameters = MatchUrlUtil.translateMatchUrl(params); 050 for (NameValuePair next : parameters) { 051 theParamValues.put(next.getName(), next.getValue()); 052 } 053 for (Map.Entry<String, Collection<String>> nextParamEntry : 054 theParamValues.asMap().entrySet()) { 055 String[] nextValue = nextParamEntry 056 .getValue() 057 .toArray(new String[nextParamEntry.getValue().size()]); 058 requestDetails.addParameter(nextParamEntry.getKey(), nextValue); 059 } 060 url = url.substring(0, qIndex); 061 } 062 063 if (url.length() > 0 && url.charAt(0) == '/') { 064 url = url.substring(1); 065 } 066 067 requestDetails.setRequestPath(url); 068 requestDetails.setFhirServerBase(theRequestDetails.getFhirServerBase()); 069 requestDetails.setTenantId(theRequestDetails.getTenantId()); 070 071 theRequestDetails.getServer().populateRequestDetailsFromRequestPath(requestDetails, url); 072 return requestDetails; 073 } 074}