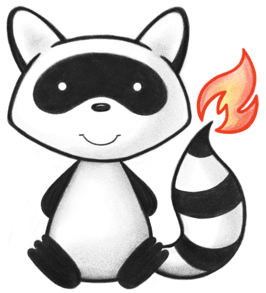
Package ca.uhn.fhir.jpa.binary.api
Interface IBinaryStorageSvc
- All Known Implementing Classes:
BaseBinaryStorageSvcImpl
,FilesystemBinaryStorageSvcImpl
,MemoryBinaryStorageSvcImpl
,NullBinaryStorageSvcImpl
public interface IBinaryStorageSvc
-
Method Summary
Modifier and TypeMethodDescriptionvoid
expungeBinaryContent
(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId) byte[]
fetchBinaryContent
(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId) Fetch the contents of the given blobfetchBinaryContentDetails
(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId) byte[]
fetchDataByteArrayFromBinary
(org.hl7.fhir.instance.model.api.IBaseBinary theResource) Fetch the byte[] contents of a given Binary resource's `data` element.long
Gets the maximum number of bytes that can be stored in a single binary file by this service.int
Gets the minimum number of bytes that will be stored.default boolean
isValidBinaryContentId
(String theNewBlobId) Given a blob ID, return true if it is valid for the underlying storage mechanism, false otherwise.Generate a new binaryContent ID that will be passed tostoreBinaryContent(IIdType, String, String, InputStream)
latervoid
setMaximumBinarySize
(long theMaximumBinarySize) Sets the maximum number of bytes that can be stored in a single binary file by this service.void
setMinimumBinarySize
(int theMinimumBinarySize) Sets the minimum number of bytes that will be stored.boolean
shouldStoreBinaryContent
(long theSize, org.hl7.fhir.instance.model.api.IIdType theResourceId, String theContentType) Give the storage service the ability to veto items from storagedefault StoredDetails
storeBinaryContent
(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobIdOrNull, String theContentType, InputStream theInputStream) Deprecated, for removal: This API element is subject to removal in a future version.storeBinaryContent
(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobIdOrNull, String theContentType, InputStream theInputStream, ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails) Store a new binary blobboolean
writeBinaryContent
(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId, OutputStream theOutputStream)
-
Method Details
-
getMaximumBinarySize
long getMaximumBinarySize()Gets the maximum number of bytes that can be stored in a single binary file by this service. The default isLong.MAX_VALUE
-
isValidBinaryContentId
Given a blob ID, return true if it is valid for the underlying storage mechanism, false otherwise.- Parameters:
theNewBlobId
- the blob ID to validate- Returns:
- true if the blob ID is valid, false otherwise.
-
setMaximumBinarySize
Sets the maximum number of bytes that can be stored in a single binary file by this service. The default isLong.MAX_VALUE
- Parameters:
theMaximumBinarySize
- The maximum size
-
getMinimumBinarySize
int getMinimumBinarySize()Gets the minimum number of bytes that will be stored. Binary content smaller * than this threshold may be inlined even if a binary storage service * is active. -
setMinimumBinarySize
Sets the minimum number of bytes that will be stored. Binary content smaller than this threshold may be inlined even if a binary storage service is active. -
shouldStoreBinaryContent
boolean shouldStoreBinaryContent(long theSize, org.hl7.fhir.instance.model.api.IIdType theResourceId, String theContentType) Give the storage service the ability to veto items from storage- Parameters:
theSize
- How large is the itemtheResourceId
- What is the resource ID it will be associated withtheContentType
- What is the content type- Returns:
true
if the storage service should store the item
-
newBinaryContentId
Generate a new binaryContent ID that will be passed tostoreBinaryContent(IIdType, String, String, InputStream)
later -
storeBinaryContent
@Deprecated(since="6.6.0", forRemoval=true) @Nonnull default StoredDetails storeBinaryContent(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobIdOrNull, String theContentType, InputStream theInputStream) throws IOException Deprecated, for removal: This API element is subject to removal in a future version.UsestoreBinaryContent(IIdType theResourceId, String theBlobIdOrNull, String theContentType, InputStream theInputStream, RequestDetails theRequestDetails)
instead. This method will be removed because it doesn't receive the 'theRequestDetails' parameter it needs to forward to the pointcut)Store a new binary blob- Parameters:
theResourceId
- The resource ID that owns this blob. Note that it should not be possible to retrieve a blob without both the resource ID and the blob ID being correct.theBlobIdOrNull
- If set, forcestheContentType
- The content type to associate with this blobtheInputStream
- An InputStream to read from. This method should close the stream when it has been fully consumed.- Returns:
- Returns details about the stored data
- Throws:
IOException
-
storeBinaryContent
@Nonnull StoredDetails storeBinaryContent(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobIdOrNull, String theContentType, InputStream theInputStream, ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails) throws IOException Store a new binary blob- Parameters:
theResourceId
- The resource ID that owns this blob. Note that it should not be possible to retrieve a blob without both the resource ID and the blob ID being correct.theBlobIdOrNull
- If set, forcestheContentType
- The content type to associate with this blobtheInputStream
- An InputStream to read from. This method should close the stream when it has been fully consumed.theRequestDetails
- The operation request details.- Returns:
- Returns details about the stored data
- Throws:
IOException
-
fetchBinaryContentDetails
StoredDetails fetchBinaryContentDetails(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId) throws IOException - Throws:
IOException
-
writeBinaryContent
boolean writeBinaryContent(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId, OutputStream theOutputStream) throws IOException - Returns:
- Returns
true
if the blob was found and written, offalse
if the blob was not found (i.e. it was expunged or the ID was invalid) - Throws:
IOException
-
expungeBinaryContent
-
fetchBinaryContent
byte[] fetchBinaryContent(org.hl7.fhir.instance.model.api.IIdType theResourceId, String theBlobId) throws IOException Fetch the contents of the given blob- Parameters:
theResourceId
- The resource IDtheBlobId
- The blob ID- Returns:
- The payload as a byte array
- Throws:
IOException
-
fetchDataByteArrayFromBinary
byte[] fetchDataByteArrayFromBinary(org.hl7.fhir.instance.model.api.IBaseBinary theResource) throws IOException Fetch the byte[] contents of a given Binary resource's `data` element. If the data is a standard base64encoded string that is embedded, return it. Otherwise, attempt to load the externalized binary blob via the the externalized binary storage service.- Parameters:
theResource
- The Binary resource you want to extract data bytes from- Returns:
- The binary data blob as a byte array
- Throws:
IOException
-
storeBinaryContent(IIdType theResourceId, String theBlobIdOrNull, String theContentType, InputStream theInputStream, RequestDetails theRequestDetails)
instead.