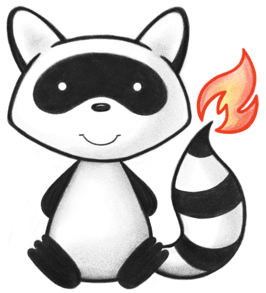
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.broker.api; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.rest.server.messaging.IMessage; 024 025/** 026 * Receives messages from a Message Broker. 027 * 028 * @param <T> The type of messages received by this consumer 029 */ 030public interface IChannelConsumer<T> extends AutoCloseable { 031 /** 032 * @return the name of the topic or queue that this consumer is consuming from 033 */ 034 String getChannelName(); 035 036 /** 037 * Start the thread(s) that will be consuming messages 038 * @throws ChannelConsumerStartFailureException if the consumer fails to start (e.g. if it fails to connect to the broker) 039 */ 040 void start(); 041 /** 042 * Close this consumer's listener and this consumer, releasing any resources. 043 */ 044 void close(); 045 046 /** 047 * @return true if this consumer is closed 048 */ 049 boolean isClosed(); 050 051 /** 052 * @return the type of messages that will be delivered to this consumer 053 */ 054 Class<? extends IMessage<T>> getMessageType(); 055 056 /** 057 * @return the {@link IMessageListener} this consumer is sending messages to (i.e. the one it was created with). 058 */ 059 IMessageListener<T> getMessageListener(); 060 061 /** 062 * Pause requesting new messages from the broker until resume() is called. 063 */ 064 default void pause() { 065 throw new UnsupportedOperationException(Msg.code(2655)); 066 } 067 068 /** 069 * Resume requesting messages from the broker. 070 */ 071 default void resume() { 072 throw new UnsupportedOperationException(Msg.code(2656)); 073 } 074 075 /** 076 * Consumers should call this method at the top of any method that attempts to use the consumer 077 */ 078 default void checkState() { 079 if (isClosed()) { 080 throw new BrokerConsumerClosedException(Msg.code(2657) + "Attempted to use a closed " 081 + this.getClass().getSimpleName() + ": " + this); 082 } 083 } 084}