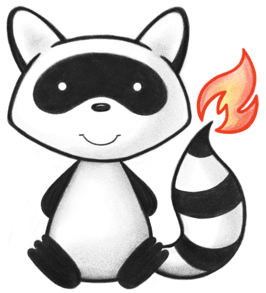
001/* 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.dao; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.support.IValidationSupport; 024import ca.uhn.fhir.rest.api.server.RequestDetails; 025import ca.uhn.fhir.util.ParametersUtil; 026import jakarta.annotation.Nonnull; 027import org.hl7.fhir.instance.model.api.IBaseCoding; 028import org.hl7.fhir.instance.model.api.IBaseDatatype; 029import org.hl7.fhir.instance.model.api.IBaseParameters; 030import org.hl7.fhir.instance.model.api.IBaseResource; 031import org.hl7.fhir.instance.model.api.IIdType; 032import org.hl7.fhir.instance.model.api.IPrimitiveType; 033import org.hl7.fhir.r4.model.codesystems.ConceptSubsumptionOutcome; 034import org.springframework.transaction.annotation.Transactional; 035 036import java.util.Collection; 037import java.util.List; 038 039public interface IFhirResourceDaoCodeSystem<T extends IBaseResource> extends IFhirResourceDao<T> { 040 041 List<IIdType> findCodeSystemIdsContainingSystemAndCode(String theCode, String theSystem, RequestDetails theRequest); 042 043 @Transactional 044 @Nonnull 045 IValidationSupport.LookupCodeResult lookupCode( 046 IPrimitiveType<String> theCode, 047 IPrimitiveType<String> theSystem, 048 IBaseCoding theCoding, 049 RequestDetails theRequestDetails); 050 051 @Nonnull 052 IValidationSupport.LookupCodeResult lookupCode( 053 IPrimitiveType<String> theCode, 054 IPrimitiveType<String> theSystem, 055 IBaseCoding theCoding, 056 IPrimitiveType<String> theDisplayLanguage, 057 RequestDetails theRequestDetails); 058 059 @Nonnull 060 IValidationSupport.LookupCodeResult lookupCode( 061 IPrimitiveType<String> theCode, 062 IPrimitiveType<String> theSystem, 063 IBaseCoding theCoding, 064 IPrimitiveType<String> theDisplayLanguage, 065 Collection<IPrimitiveType<String>> thePropertyNames, 066 RequestDetails theRequestDetails); 067 068 SubsumesResult subsumes( 069 IPrimitiveType<String> theCodeA, 070 IPrimitiveType<String> theCodeB, 071 IPrimitiveType<String> theSystem, 072 IBaseCoding theCodingA, 073 IBaseCoding theCodingB, 074 RequestDetails theRequestDetails); 075 076 @Nonnull 077 IValidationSupport.CodeValidationResult validateCode( 078 IIdType theCodeSystemId, 079 IPrimitiveType<String> theCodeSystemUrl, 080 IPrimitiveType<String> theVersion, 081 IPrimitiveType<String> theCode, 082 IPrimitiveType<String> theDisplay, 083 IBaseCoding theCoding, 084 IBaseDatatype theCodeableConcept, 085 RequestDetails theRequestDetails); 086 087 class SubsumesResult { 088 089 private final ConceptSubsumptionOutcome myOutcome; 090 091 public SubsumesResult(ConceptSubsumptionOutcome theOutcome) { 092 myOutcome = theOutcome; 093 } 094 095 public ConceptSubsumptionOutcome getOutcome() { 096 return myOutcome; 097 } 098 099 @SuppressWarnings("unchecked") 100 public IBaseParameters toParameters(FhirContext theFhirContext) { 101 IBaseParameters retVal = ParametersUtil.newInstance(theFhirContext); 102 103 IPrimitiveType<String> outcomeValue = (IPrimitiveType<String>) 104 theFhirContext.getElementDefinition("code").newInstance(); 105 outcomeValue.setValueAsString(getOutcome().toCode()); 106 ParametersUtil.addParameterToParameters(theFhirContext, retVal, "outcome", outcomeValue); 107 108 return retVal; 109 } 110 } 111}