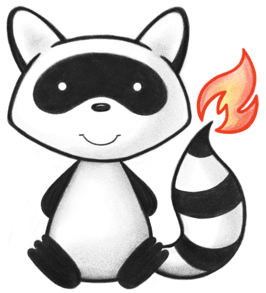
001/* 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.dao; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.api.model.ExpungeOptions; 024import ca.uhn.fhir.jpa.api.model.ExpungeOutcome; 025import ca.uhn.fhir.rest.api.server.IBundleProvider; 026import ca.uhn.fhir.rest.api.server.RequestDetails; 027import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 028import jakarta.annotation.Nullable; 029import org.hl7.fhir.instance.model.api.IBaseBundle; 030import org.springframework.transaction.annotation.Transactional; 031 032import java.util.Date; 033import java.util.List; 034import java.util.Map; 035 036/** 037 * Note that this interface is not considered a stable interface. While it is possible to build applications 038 * that use it directly, please be aware that we may modify methods, add methods, or even remove methods from 039 * time to time, even within minor point releases. 040 * 041 * @param <T> The bundle type 042 * @param <MT> The Meta datatype type 043 */ 044public interface IFhirSystemDao<T, MT> extends IDao { 045 046 ExpungeOutcome expunge(ExpungeOptions theExpungeOptions, RequestDetails theRequestDetails); 047 048 Map<String, Long> getResourceCounts(); 049 050 /** 051 * Returns a cached count of resources using a cache that regularly 052 * refreshes in the background. This method will never block, and may return null if nothing is in the cache. 053 */ 054 @Nullable 055 Map<String, Long> getResourceCountsFromCache(); 056 057 IBundleProvider history(Date theDate, Date theUntil, Integer theOffset, RequestDetails theRequestDetails); 058 059 /** 060 * Not supported for DSTU1 061 * 062 * @param theRequestDetails TODO 063 */ 064 @Transactional 065 MT metaGetOperation(RequestDetails theRequestDetails); 066 067 /** 068 * Implementations may implement this method to implement the $process-message 069 * operation 070 */ 071 IBaseBundle processMessage(RequestDetails theRequestDetails, IBaseBundle theMessage); 072 073 /** 074 * Executes a FHIR transaction using a new database transaction. This method must 075 * not be called from within a DB transaction. 076 */ 077 T transaction(RequestDetails theRequestDetails, T theResources); 078 079 /** 080 * Executes a FHIR transaction nested inside the current database transaction. 081 * This form of the transaction processor can handle write operations only (no reads) 082 */ 083 default T transactionNested(RequestDetails theRequestDetails, T theResources) { 084 throw new UnsupportedOperationException(Msg.code(570)); 085 } 086 087 /** 088 * Preload resources from the database in batch. This method is purely 089 * a performance optimization and must be purely idempotent. 090 * 091 * @param thePreFetchIndexes Should resource indexes be loaded 092 */ 093 default <P extends IResourcePersistentId> void preFetchResources( 094 List<P> theResolvedIds, boolean thePreFetchIndexes) { 095 // nothing by default 096 } 097}