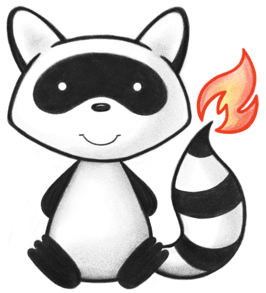
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.dao; 021 022import ca.uhn.fhir.model.api.annotation.Description; 023import ca.uhn.fhir.rest.api.SortSpec; 024import ca.uhn.fhir.rest.param.DateRangeParam; 025import ca.uhn.fhir.rest.param.StringAndListParam; 026import org.hl7.fhir.instance.model.api.IPrimitiveType; 027 028public final class PatientEverythingParameters { 029 @Description( 030 formalDefinition = 031 "Results from this method are returned across multiple pages. This parameter controls the size of those pages.") 032 private IPrimitiveType<Integer> myCount; 033 034 @Description( 035 formalDefinition = 036 "Results from this method are returned across multiple pages. This parameter controls the offset when fetching a page.") 037 private IPrimitiveType<Integer> myOffset; 038 039 @Description(shortDefinition = "Only return resources which were last updated as specified by the given range") 040 private DateRangeParam myLastUpdated; 041 042 @Description(shortDefinition = "The order in which to sort the results by") 043 private SortSpec mySort; 044 045 @Description( 046 shortDefinition = 047 "Filter the resources to return only resources matching the given _content filter (note that this filter is applied only to results which link to the given patient, not to the patient itself or to supporting resources linked to by the matched resources)") 048 private StringAndListParam myContent; 049 050 @Description( 051 shortDefinition = 052 "Filter the resources to return only resources matching the given _text filter (note that this filter is applied only to results which link to the given patient, not to the patient itself or to supporting resources linked to by the matched resources)") 053 private StringAndListParam myNarrative; 054 055 @Description( 056 shortDefinition = 057 "Filter the resources to return only resources matching the given _filter filter (note that this filter is applied only to results which link to the given patient, not to the patient itself or to supporting resources linked to by the matched resources)") 058 private StringAndListParam myFilter; 059 060 @Description( 061 shortDefinition = 062 "Filter the resources to return only resources matching the given _type filter (note that this filter is applied only to results which link to the given patient, not to the patient itself or to supporting resources linked to by the matched resources)") 063 private StringAndListParam myTypes; 064 065 @Description( 066 shortDefinition = "If set to true, trigger an MDM expansion of identifiers corresponding to the resources.") 067 private boolean myMdmExpand = false; 068 069 public IPrimitiveType<Integer> getCount() { 070 return myCount; 071 } 072 073 public IPrimitiveType<Integer> getOffset() { 074 return myOffset; 075 } 076 077 public DateRangeParam getLastUpdated() { 078 return myLastUpdated; 079 } 080 081 public SortSpec getSort() { 082 return mySort; 083 } 084 085 public StringAndListParam getContent() { 086 return myContent; 087 } 088 089 public StringAndListParam getNarrative() { 090 return myNarrative; 091 } 092 093 public StringAndListParam getFilter() { 094 return myFilter; 095 } 096 097 public StringAndListParam getTypes() { 098 return myTypes; 099 } 100 101 public boolean getMdmExpand() { 102 return myMdmExpand; 103 } 104 105 public void setCount(IPrimitiveType<Integer> theCount) { 106 this.myCount = theCount; 107 } 108 109 public void setOffset(IPrimitiveType<Integer> theOffset) { 110 this.myOffset = theOffset; 111 } 112 113 public void setLastUpdated(DateRangeParam theLastUpdated) { 114 this.myLastUpdated = theLastUpdated; 115 } 116 117 public void setSort(SortSpec theSort) { 118 this.mySort = theSort; 119 } 120 121 public void setContent(StringAndListParam theContent) { 122 this.myContent = theContent; 123 } 124 125 public void setNarrative(StringAndListParam theNarrative) { 126 this.myNarrative = theNarrative; 127 } 128 129 public void setFilter(StringAndListParam theFilter) { 130 this.myFilter = theFilter; 131 } 132 133 public void setTypes(StringAndListParam theTypes) { 134 this.myTypes = theTypes; 135 } 136 137 public void setMdmExpand(Boolean myMdmExpand) { 138 this.myMdmExpand = myMdmExpand; 139 } 140}