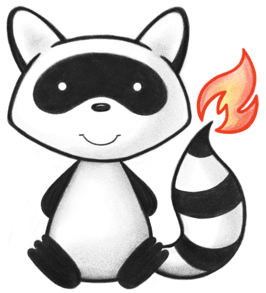
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import ca.uhn.fhir.model.api.IModelJson; 023import com.fasterxml.jackson.annotation.JsonProperty; 024 025import java.util.HashMap; 026import java.util.List; 027import java.util.Map; 028 029public class BulkExportJobResults implements IModelJson { 030 031 @JsonProperty("resourceType2BinaryIds") 032 private Map<String, List<String>> myResourceTypeToBinaryIds; 033 034 @JsonProperty("reportMessage") 035 private String myReportMsg; 036 037 @JsonProperty("originalRequestUrl") 038 private String myOriginalRequestUrl; 039 040 public BulkExportJobResults() {} 041 042 public Map<String, List<String>> getResourceTypeToBinaryIds() { 043 if (myResourceTypeToBinaryIds == null) { 044 myResourceTypeToBinaryIds = new HashMap<>(); 045 } 046 return myResourceTypeToBinaryIds; 047 } 048 049 public String getOriginalRequestUrl() { 050 return myOriginalRequestUrl; 051 } 052 053 public void setOriginalRequestUrl(String theOriginalRequestUrl) { 054 myOriginalRequestUrl = theOriginalRequestUrl; 055 } 056 057 public void setResourceTypeToBinaryIds(Map<String, List<String>> theResourceTypeToBinaryIds) { 058 myResourceTypeToBinaryIds = theResourceTypeToBinaryIds; 059 } 060 061 public String getReportMsg() { 062 return myReportMsg; 063 } 064 065 public void setReportMsg(String theReportMsg) { 066 myReportMsg = theReportMsg; 067 } 068}