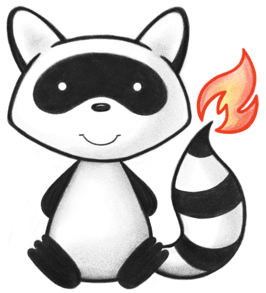
001/* 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import ca.uhn.fhir.jpa.model.cross.IBasePersistedResource; 023import ca.uhn.fhir.rest.api.MethodOutcome; 024import ca.uhn.fhir.rest.api.RestOperationTypeEnum; 025import ca.uhn.fhir.rest.api.server.storage.IResourcePersistentId; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027 028public class DaoMethodOutcome extends MethodOutcome { 029 030 private IBasePersistedResource myEntity; 031 private IBaseResource myPreviousResource; 032 private boolean myNop; 033 private IResourcePersistentId myResourcePersistentId; 034 private RestOperationTypeEnum myOperationType; 035 private String myMatchUrl; 036 037 /** 038 * Constructor 039 */ 040 public DaoMethodOutcome() { 041 super(); 042 } 043 044 public RestOperationTypeEnum getOperationType() { 045 return myOperationType; 046 } 047 048 public void setOperationType(RestOperationTypeEnum theOperationType) { 049 myOperationType = theOperationType; 050 } 051 052 public String getMatchUrl() { 053 return myMatchUrl; 054 } 055 056 public void setMatchUrl(String theMatchUrl) { 057 myMatchUrl = theMatchUrl; 058 } 059 060 /** 061 * Was this a NO-OP - Typically because of an update to a resource that already matched the contents provided 062 */ 063 public boolean isNop() { 064 return myNop; 065 } 066 067 /** 068 * Was this a NO-OP - Typically because of an update to a resource that already matched the contents provided 069 */ 070 public DaoMethodOutcome setNop(boolean theNop) { 071 myNop = theNop; 072 return this; 073 } 074 075 public IBasePersistedResource getEntity() { 076 return myEntity; 077 } 078 079 public DaoMethodOutcome setEntity(IBasePersistedResource theEntity) { 080 myEntity = theEntity; 081 return this; 082 } 083 084 /** 085 * For update operations, this is the body of the resource as it was before the 086 * update 087 */ 088 public IBaseResource getPreviousResource() { 089 return myPreviousResource; 090 } 091 092 /** 093 * For update operations, this is the body of the resource as it was before the 094 * update 095 */ 096 public void setPreviousResource(IBaseResource thePreviousResource) { 097 myPreviousResource = thePreviousResource; 098 } 099 100 @Override 101 public DaoMethodOutcome setCreated(Boolean theCreated) { 102 super.setCreated(theCreated); 103 return this; 104 } 105 106 public IResourcePersistentId getPersistentId() { 107 return myResourcePersistentId; 108 } 109 110 public DaoMethodOutcome setPersistentId(IResourcePersistentId theResourcePersistentId) { 111 myResourcePersistentId = theResourcePersistentId; 112 return this; 113 } 114}