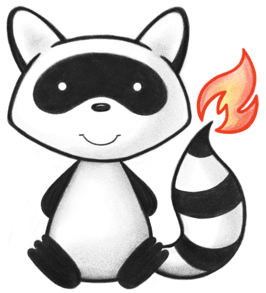
001/* 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import ca.uhn.fhir.model.primitive.IdDt; 023import org.apache.commons.lang3.builder.ToStringBuilder; 024import org.apache.commons.lang3.builder.ToStringStyle; 025 026public class DeleteConflict { 027 028 private final IdDt mySourceId; 029 private final String mySourcePath; 030 private final IdDt myTargetId; 031 032 public DeleteConflict(IdDt theSourceId, String theSourcePath, IdDt theTargetId) { 033 mySourceId = theSourceId; 034 mySourcePath = theSourcePath; 035 myTargetId = theTargetId; 036 } 037 038 public IdDt getSourceId() { 039 return mySourceId; 040 } 041 042 public String getSourcePath() { 043 return mySourcePath; 044 } 045 046 public IdDt getTargetId() { 047 return myTargetId; 048 } 049 050 @Override 051 public String toString() { 052 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 053 .append("sourceId", mySourceId) 054 .append("sourcePath", mySourcePath) 055 .append("targetId", myTargetId) 056 .toString(); 057 } 058}