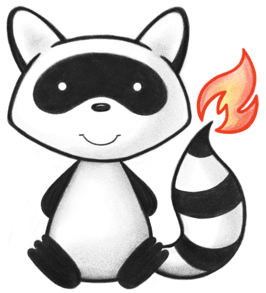
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import org.apache.commons.lang3.builder.ToStringBuilder; 023import org.apache.commons.lang3.builder.ToStringStyle; 024 025public class ExpungeOptions { 026 private int myLimit = 1000; 027 private boolean myExpungeOldVersions; 028 private boolean myExpungeDeletedResources; 029 private boolean myExpungeEverything; 030 031 @Override 032 public String toString() { 033 return new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE) 034 .append("limit", myLimit) 035 .append("oldVersions", myExpungeOldVersions) 036 .append("deletedResources", myExpungeDeletedResources) 037 .append("everything", myExpungeEverything) 038 .toString(); 039 } 040 041 /** 042 * The maximum number of resources versions to expunge 043 */ 044 public int getLimit() { 045 return myLimit; 046 } 047 048 /** 049 * The maximum number of resource versions to expunge 050 */ 051 public ExpungeOptions setLimit(int theLimit) { 052 myLimit = theLimit; 053 return this; 054 } 055 056 public boolean isExpungeEverything() { 057 return myExpungeEverything; 058 } 059 060 public ExpungeOptions setExpungeEverything(boolean theExpungeEverything) { 061 myExpungeEverything = theExpungeEverything; 062 return this; 063 } 064 065 public boolean isExpungeDeletedResources() { 066 return myExpungeDeletedResources; 067 } 068 069 public ExpungeOptions setExpungeDeletedResources(boolean theExpungeDeletedResources) { 070 myExpungeDeletedResources = theExpungeDeletedResources; 071 return this; 072 } 073 074 public boolean isExpungeOldVersions() { 075 return myExpungeOldVersions; 076 } 077 078 public ExpungeOptions setExpungeOldVersions(boolean theExpungeOldVersions) { 079 myExpungeOldVersions = theExpungeOldVersions; 080 return this; 081 } 082}