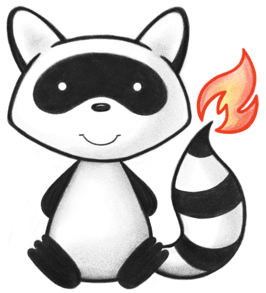
001/* 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import org.apache.commons.lang3.builder.EqualsBuilder; 023import org.apache.commons.lang3.builder.HashCodeBuilder; 024import org.hl7.fhir.instance.model.api.IIdType; 025import org.hl7.fhir.r4.model.Coding; 026 027import static org.apache.commons.lang3.StringUtils.isNotBlank; 028 029public class TranslationQuery { 030 private Coding myCoding; 031 private IIdType myResourceId; 032 private String myUrl; 033 private String myConceptMapVersion; 034 private String mySource; 035 private String myTarget; 036 private String myTargetSystem; 037 038 public TranslationQuery() { 039 super(); 040 041 myCoding = new Coding(); 042 } 043 044 public Coding getCoding() { 045 return myCoding; 046 } 047 048 public void setCoding(Coding theCoding) { 049 myCoding = theCoding; 050 } 051 052 public boolean hasResourceId() { 053 return myResourceId != null; 054 } 055 056 public IIdType getResourceId() { 057 return myResourceId; 058 } 059 060 public void setResourceId(IIdType theResourceId) { 061 myResourceId = theResourceId; 062 } 063 064 public boolean hasUrl() { 065 return isNotBlank(myUrl); 066 } 067 068 public String getUrl() { 069 return myUrl; 070 } 071 072 public void setUrl(String theUrl) { 073 myUrl = theUrl; 074 } 075 076 public boolean hasConceptMapVersion() { 077 return isNotBlank(myConceptMapVersion); 078 } 079 080 public String getConceptMapVersion() { 081 return myConceptMapVersion; 082 } 083 084 public void setConceptMapVersion(String theConceptMapVersion) { 085 myConceptMapVersion = theConceptMapVersion; 086 } 087 088 public boolean hasSource() { 089 return isNotBlank(mySource); 090 } 091 092 public String getSource() { 093 return mySource; 094 } 095 096 public void setSource(String theSource) { 097 mySource = theSource; 098 } 099 100 public boolean hasTarget() { 101 return isNotBlank(myTarget); 102 } 103 104 public String getTarget() { 105 return myTarget; 106 } 107 108 public void setTarget(String theTarget) { 109 myTarget = theTarget; 110 } 111 112 public boolean hasTargetSystem() { 113 return isNotBlank(myTargetSystem); 114 } 115 116 public String getTargetSystem() { 117 return myTargetSystem; 118 } 119 120 public void setTargetSystem(String theTargetSystem) { 121 myTargetSystem = theTargetSystem; 122 } 123 124 @Override 125 public boolean equals(Object o) { 126 if (this == o) return true; 127 128 if (!(o instanceof TranslationQuery)) return false; 129 130 TranslationQuery that = (TranslationQuery) o; 131 132 return new EqualsBuilder() 133 .append(getCoding().getCode(), that.getCoding().getCode()) 134 .append(getCoding().getSystem(), that.getCoding().getSystem()) 135 .append(getCoding().getVersion(), that.getCoding().getVersion()) 136 .append(getResourceId(), that.getResourceId()) 137 .append(getUrl(), that.getUrl()) 138 .append(getConceptMapVersion(), that.getConceptMapVersion()) 139 .append(getSource(), that.getSource()) 140 .append(getTarget(), that.getTarget()) 141 .append(getTargetSystem(), that.getTargetSystem()) 142 .isEquals(); 143 } 144 145 @Override 146 public int hashCode() { 147 return new HashCodeBuilder(17, 37) 148 .append(getCoding().getCode()) 149 .append(getCoding().getSystem()) 150 .append(getCoding().getVersion()) 151 .append(getResourceId()) 152 .append(getUrl()) 153 .append(getConceptMapVersion()) 154 .append(getSource()) 155 .append(getTarget()) 156 .append(getTargetSystem()) 157 .toHashCode(); 158 } 159}