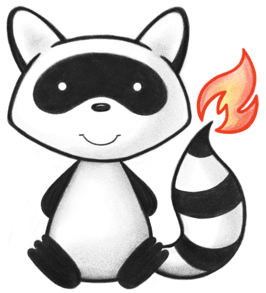
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import ca.uhn.fhir.context.support.IValidationSupport; 023import org.apache.commons.lang3.Validate; 024import org.hl7.fhir.instance.model.api.IBaseCoding; 025import org.hl7.fhir.instance.model.api.IIdType; 026import org.hl7.fhir.r4.model.CodeableConcept; 027import org.hl7.fhir.r4.model.Coding; 028 029import java.util.ArrayList; 030import java.util.Collections; 031import java.util.List; 032 033import static org.apache.commons.lang3.StringUtils.isNotBlank; 034 035public class TranslationRequest { 036 private CodeableConcept myCodeableConcept; 037 private IIdType myResourceId; 038 private Boolean myReverse; 039 private String myUrl; 040 private String myConceptMapVersion; 041 private String mySource; 042 private String myTarget; 043 private String myTargetSystem; 044 045 public TranslationRequest() { 046 super(); 047 048 myCodeableConcept = new CodeableConcept(); 049 } 050 051 /** 052 * This is just a convenience method that creates a codeableconcept if one 053 * doesn't already exist, and adds a coding to it 054 */ 055 public TranslationRequest addCode(String theSystem, String theCode) { 056 Validate.notBlank(theSystem, "theSystem must not be null"); 057 Validate.notBlank(theCode, "theCode must not be null"); 058 if (getCodeableConcept() == null) { 059 setCodeableConcept(new CodeableConcept()); 060 } 061 getCodeableConcept().addCoding(new Coding().setSystem(theSystem).setCode(theCode)); 062 return this; 063 } 064 065 public CodeableConcept getCodeableConcept() { 066 return myCodeableConcept; 067 } 068 069 public TranslationRequest setCodeableConcept(CodeableConcept theCodeableConcept) { 070 myCodeableConcept = theCodeableConcept; 071 return this; 072 } 073 074 public IIdType getResourceId() { 075 return myResourceId; 076 } 077 078 public void setResourceId(IIdType theResourceId) { 079 myResourceId = theResourceId; 080 } 081 082 public Boolean getReverse() { 083 return myReverse; 084 } 085 086 public void setReverse(Boolean theReverse) { 087 myReverse = theReverse; 088 } 089 090 public boolean getReverseAsBoolean() { 091 if (hasReverse()) { 092 return myReverse; 093 } 094 095 return false; 096 } 097 098 public String getUrl() { 099 return myUrl; 100 } 101 102 public TranslationRequest setUrl(String theUrl) { 103 myUrl = theUrl; 104 return this; 105 } 106 107 public String getConceptMapVersion() { 108 return myConceptMapVersion; 109 } 110 111 public TranslationRequest setConceptMapVersion(String theConceptMapVersion) { 112 myConceptMapVersion = theConceptMapVersion; 113 return this; 114 } 115 116 public String getSource() { 117 return mySource; 118 } 119 120 public TranslationRequest setSource(String theSource) { 121 mySource = theSource; 122 return this; 123 } 124 125 public String getTarget() { 126 return myTarget; 127 } 128 129 public TranslationRequest setTarget(String theTarget) { 130 myTarget = theTarget; 131 return this; 132 } 133 134 public String getTargetSystem() { 135 return myTargetSystem; 136 } 137 138 public TranslationRequest setTargetSystem(String theTargetSystem) { 139 myTargetSystem = theTargetSystem; 140 return this; 141 } 142 143 public List<TranslationQuery> getTranslationQueries() { 144 List<TranslationQuery> retVal = new ArrayList<>(); 145 146 TranslationQuery translationQuery; 147 for (Coding coding : getCodeableConcept().getCoding()) { 148 translationQuery = new TranslationQuery(); 149 150 translationQuery.setCoding(coding); 151 152 if (this.hasResourceId()) { 153 translationQuery.setResourceId(this.getResourceId()); 154 } 155 156 if (this.hasUrl()) { 157 translationQuery.setUrl(this.getUrl()); 158 } 159 160 if (this.hasConceptMapVersion()) { 161 translationQuery.setConceptMapVersion(this.getConceptMapVersion()); 162 } 163 164 if (this.hasSource()) { 165 translationQuery.setSource(this.getSource()); 166 } 167 168 if (this.hasTarget()) { 169 translationQuery.setTarget(this.getTarget()); 170 } 171 172 if (this.hasTargetSystem()) { 173 translationQuery.setTargetSystem(this.getTargetSystem()); 174 } 175 176 retVal.add(translationQuery); 177 } 178 179 return retVal; 180 } 181 182 public boolean hasResourceId() { 183 return myResourceId != null; 184 } 185 186 public boolean hasReverse() { 187 return myReverse != null; 188 } 189 190 public boolean hasUrl() { 191 return isNotBlank(myUrl); 192 } 193 194 public boolean hasConceptMapVersion() { 195 return isNotBlank(myConceptMapVersion); 196 } 197 198 public boolean hasSource() { 199 return isNotBlank(mySource); 200 } 201 202 public boolean hasTarget() { 203 return isNotBlank(myTarget); 204 } 205 206 public boolean hasTargetSystem() { 207 return isNotBlank(myTargetSystem); 208 } 209 210 public IValidationSupport.TranslateCodeRequest asTranslateCodeRequest() { 211 return new IValidationSupport.TranslateCodeRequest( 212 Collections.unmodifiableList(this.getCodeableConcept().getCoding()), 213 this.getTargetSystem(), 214 this.getUrl(), 215 this.getConceptMapVersion(), 216 this.getSource(), 217 this.getTarget(), 218 this.getResourceId(), 219 this.getReverseAsBoolean()); 220 } 221 222 public static TranslationRequest fromTranslateCodeRequest(IValidationSupport.TranslateCodeRequest theRequest) { 223 CodeableConcept sourceCodeableConcept = new CodeableConcept(); 224 for (IBaseCoding aCoding : theRequest.getCodings()) { 225 sourceCodeableConcept 226 .addCoding() 227 .setSystem(aCoding.getSystem()) 228 .setCode(aCoding.getCode()) 229 .setVersion(((Coding) aCoding).getVersion()); 230 } 231 232 TranslationRequest translationRequest = new TranslationRequest(); 233 translationRequest.setCodeableConcept(sourceCodeableConcept); 234 translationRequest.setConceptMapVersion(theRequest.getConceptMapVersion()); 235 translationRequest.setUrl(theRequest.getConceptMapUrl()); 236 translationRequest.setSource(theRequest.getSourceValueSetUrl()); 237 translationRequest.setTarget(theRequest.getTargetValueSetUrl()); 238 translationRequest.setTargetSystem(theRequest.getTargetSystemUrl()); 239 translationRequest.setResourceId(theRequest.getResourceId()); 240 translationRequest.setReverse(theRequest.isReverse()); 241 return translationRequest; 242 } 243}