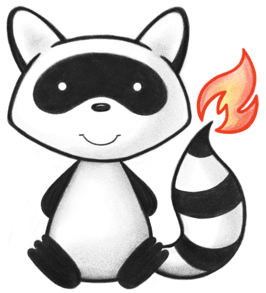
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.model; 021 022import org.apache.commons.lang3.builder.EqualsBuilder; 023import org.apache.commons.lang3.builder.HashCodeBuilder; 024 025/** 026 * Denotes a search that should be performed in the background 027 * periodically in order to keep a fresh copy in the query cache. 028 * This improves performance for searches by keeping a copy 029 * loaded in the background. 030 */ 031public class WarmCacheEntry { 032 033 private long myPeriodMillis; 034 private String myUrl; 035 036 @Override 037 public boolean equals(Object theO) { 038 if (this == theO) { 039 return true; 040 } 041 042 if (theO == null || getClass() != theO.getClass()) { 043 return false; 044 } 045 046 WarmCacheEntry that = (WarmCacheEntry) theO; 047 048 return new EqualsBuilder() 049 .append(myPeriodMillis, that.myPeriodMillis) 050 .append(myUrl, that.myUrl) 051 .isEquals(); 052 } 053 054 @Override 055 public int hashCode() { 056 return new HashCodeBuilder(17, 37).append(myPeriodMillis).append(myUrl).toHashCode(); 057 } 058 059 public long getPeriodMillis() { 060 return myPeriodMillis; 061 } 062 063 public WarmCacheEntry setPeriodMillis(long thePeriodMillis) { 064 myPeriodMillis = thePeriodMillis; 065 return this; 066 } 067 068 public String getUrl() { 069 return myUrl; 070 } 071 072 public WarmCacheEntry setUrl(String theUrl) { 073 myUrl = theUrl; 074 return this; 075 } 076}