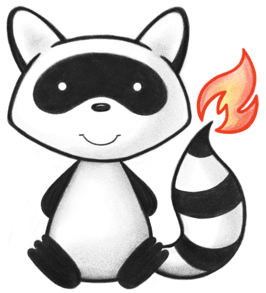
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.api.svc; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.interceptor.model.RequestPartitionId; 024import ca.uhn.fhir.jpa.api.pid.IResourcePidList; 025import ca.uhn.fhir.jpa.api.pid.IResourcePidStream; 026import ca.uhn.fhir.jpa.api.pid.ListWrappingPidStream; 027import ca.uhn.fhir.model.primitive.IdDt; 028import jakarta.annotation.Nonnull; 029import jakarta.annotation.Nullable; 030import org.hl7.fhir.instance.model.api.IIdType; 031 032import java.util.Date; 033import java.util.stream.Stream; 034 035public interface IBatch2DaoSvc { 036 037 /** 038 * Indicates whether reindexing all resource types is supported. Implementations are expected to provide a static response (either they support this or they don't). 039 */ 040 boolean isAllResourceTypeSupported(); 041 042 /** 043 * Fetches a page of resource IDs for all resource types. The page size is up to the discretion of the implementation. 044 * 045 * @param theStart The start of the date range, must be inclusive. 046 * @param theEnd The end of the date range, should be exclusive. 047 * @param theRequestPartitionId The request partition ID (may be <code>null</code> on non-partitioned systems) 048 * @param theUrl The search URL, or <code>null</code> to return IDs for all resources across all resource types. Null will only be supplied if {@link #isAllResourceTypeSupported()} returns <code>true</code>. 049 */ 050 default IResourcePidList fetchResourceIdsPage( 051 Date theStart, Date theEnd, @Nullable RequestPartitionId theRequestPartitionId, @Nullable String theUrl) { 052 throw new UnsupportedOperationException(Msg.code(2425) + "Not implemented unless explicitly overridden"); 053 } 054 055 // TODO: LD: eliminate this call in all other implementors 056 /** 057 * @deprecated Please call (@link {@link #fetchResourceIdsPage(Date, Date, RequestPartitionId, String)} instead. 058 * <p/> 059 * Fetches a page of resource IDs for all resource types. The page size is up to the discretion of the implementation. 060 * 061 * @param theStart The start of the date range, must be inclusive. 062 * @param theEnd The end of the date range, should be exclusive. 063 * @param thePageSize The number of records to query in each pass. 064 * @param theRequestPartitionId The request partition ID (may be <code>null</code> on non-partitioned systems) 065 * @param theUrl The search URL, or <code>null</code> to return IDs for all resources across all resource types. Null will only be supplied if {@link #isAllResourceTypeSupported()} returns <code>true</code>. 066 */ 067 @Deprecated 068 default IResourcePidList fetchResourceIdsPage( 069 Date theStart, 070 Date theEnd, 071 @Nonnull Integer thePageSize, 072 @Nullable RequestPartitionId theRequestPartitionId, 073 @Nullable String theUrl) { 074 return fetchResourceIdsPage(theStart, theEnd, theRequestPartitionId, theUrl); 075 } 076 077 default IResourcePidStream fetchResourceIdStream( 078 Date theStart, Date theEnd, RequestPartitionId theTargetPartitionId, String theUrl) { 079 return new ListWrappingPidStream(fetchResourceIdsPage( 080 theStart, theEnd, 20000 /* ResourceIdListStep.DEFAULT_PAGE_SIZE */, theTargetPartitionId, theUrl)); 081 } 082 083 /** 084 * Stream Resource Ids of all resources that have a reference to the provided resource id 085 * 086 * @param theTargetId the id of the resource we are searching for references to 087 */ 088 default Stream<IdDt> streamSourceIdsThatReferenceTargetId(IIdType theTargetId) { 089 throw new UnsupportedOperationException(Msg.code(2594) + "Not implemented unless explicitly overridden"); 090 } 091}