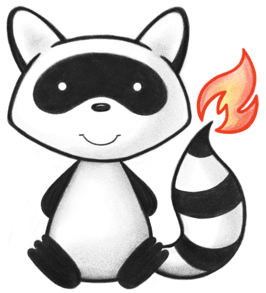
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.binary.api; 021 022import ca.uhn.fhir.model.api.IModelJson; 023import ca.uhn.fhir.rest.server.util.JsonDateDeserializer; 024import ca.uhn.fhir.rest.server.util.JsonDateSerializer; 025import com.fasterxml.jackson.annotation.JsonProperty; 026import com.fasterxml.jackson.databind.annotation.JsonDeserialize; 027import com.fasterxml.jackson.databind.annotation.JsonSerialize; 028import com.google.common.hash.HashingInputStream; 029import jakarta.annotation.Nonnull; 030import org.apache.commons.lang3.builder.ToStringBuilder; 031 032import java.util.Date; 033 034public class StoredDetails implements IModelJson { 035 036 @JsonProperty("binaryContentId") 037 private String myBinaryContentId; 038 039 @JsonProperty("bytes") 040 private long myBytes; 041 042 @JsonProperty("contentType") 043 private String myContentType; 044 045 @JsonProperty("hash") 046 private String myHash; 047 048 @JsonProperty("published") 049 @JsonSerialize(using = JsonDateSerializer.class) 050 @JsonDeserialize(using = JsonDateDeserializer.class) 051 private Date myPublished; 052 053 /** 054 * Constructor 055 */ 056 @SuppressWarnings("unused") 057 public StoredDetails() { 058 super(); 059 } 060 061 /** 062 * Constructor 063 */ 064 public StoredDetails( 065 @Nonnull String theBinaryContentId, 066 long theBytes, 067 @Nonnull String theContentType, 068 HashingInputStream theIs, 069 Date thePublished) { 070 myBinaryContentId = theBinaryContentId; 071 myBytes = theBytes; 072 myContentType = theContentType; 073 myHash = theIs.hash().toString(); 074 myPublished = thePublished; 075 } 076 077 @Override 078 public String toString() { 079 return new ToStringBuilder(this) 080 .append("binaryContentId", myBinaryContentId) 081 .append("bytes", myBytes) 082 .append("contentType", myContentType) 083 .append("hash", myHash) 084 .append("published", myPublished) 085 .toString(); 086 } 087 088 public String getHash() { 089 return myHash; 090 } 091 092 public StoredDetails setHash(String theHash) { 093 myHash = theHash; 094 return this; 095 } 096 097 public Date getPublished() { 098 return myPublished; 099 } 100 101 public StoredDetails setPublished(Date thePublished) { 102 myPublished = thePublished; 103 return this; 104 } 105 106 @Nonnull 107 public String getContentType() { 108 return myContentType; 109 } 110 111 public StoredDetails setContentType(String theContentType) { 112 myContentType = theContentType; 113 return this; 114 } 115 116 @Nonnull 117 public String getBinaryContentId() { 118 return myBinaryContentId; 119 } 120 121 public StoredDetails setBinaryContentId(String theBinaryContentId) { 122 myBinaryContentId = theBinaryContentId; 123 return this; 124 } 125 126 public long getBytes() { 127 return myBytes; 128 } 129 130 public StoredDetails setBytes(long theBytes) { 131 myBytes = theBytes; 132 return this; 133 } 134}