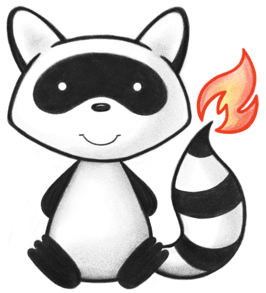
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.binary.svc; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.jpa.binary.api.IBinaryStorageSvc; 024import ca.uhn.fhir.jpa.binary.api.StoredDetails; 025import ca.uhn.fhir.rest.api.server.RequestDetails; 026import jakarta.annotation.Nonnull; 027import org.hl7.fhir.instance.model.api.IBaseBinary; 028import org.hl7.fhir.instance.model.api.IIdType; 029 030import java.io.InputStream; 031import java.io.OutputStream; 032 033public class NullBinaryStorageSvcImpl implements IBinaryStorageSvc { 034 035 @Override 036 public long getMaximumBinarySize() { 037 return 0; 038 } 039 040 @Override 041 public boolean isValidBinaryContentId(String theNewBlobId) { 042 return true; 043 } 044 045 @Override 046 public void setMaximumBinarySize(long theMaximumBinarySize) { 047 // ignore 048 } 049 050 @Override 051 public int getMinimumBinarySize() { 052 return 0; 053 } 054 055 @Override 056 public void setMinimumBinarySize(int theMinimumBinarySize) { 057 // ignore 058 } 059 060 @Override 061 public boolean shouldStoreBinaryContent(long theSize, IIdType theResourceId, String theContentType) { 062 return false; 063 } 064 065 @Override 066 public String newBinaryContentId() { 067 throw new UnsupportedOperationException(Msg.code(1345)); 068 } 069 070 @Nonnull 071 @Override 072 public StoredDetails storeBinaryContent( 073 IIdType theResourceId, 074 String theBlobIdOrNull, 075 String theContentType, 076 InputStream theInputStream, 077 RequestDetails theRequestDetails) { 078 throw new UnsupportedOperationException(Msg.code(1346)); 079 } 080 081 @Override 082 public StoredDetails fetchBinaryContentDetails(IIdType theResourceId, String theBlobId) { 083 throw new UnsupportedOperationException(Msg.code(1347)); 084 } 085 086 @Override 087 public boolean writeBinaryContent(IIdType theResourceId, String theBlobId, OutputStream theOutputStream) { 088 throw new UnsupportedOperationException(Msg.code(1348)); 089 } 090 091 @Override 092 public void expungeBinaryContent(IIdType theIdElement, String theBlobId) { 093 throw new UnsupportedOperationException(Msg.code(1349)); 094 } 095 096 @Override 097 public byte[] fetchBinaryContent(IIdType theResourceId, String theBlobId) { 098 throw new UnsupportedOperationException(Msg.code(1350)); 099 } 100 101 @Override 102 public byte[] fetchDataByteArrayFromBinary(IBaseBinary theResource) { 103 throw new UnsupportedOperationException(Msg.code(1351)); 104 } 105}