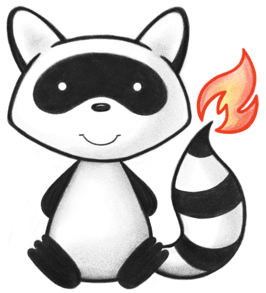
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.bulk.imprt.api; 021 022import ca.uhn.fhir.jpa.bulk.imprt.model.ActivateJobResult; 023import ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobFileJson; 024import ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobJson; 025import ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum; 026import jakarta.annotation.Nonnull; 027 028import java.util.Date; 029import java.util.List; 030 031public interface IBulkDataImportSvc { 032 033 class JobInfo { 034 private BulkImportJobStatusEnum myStatus; 035 private Date myStatusTime; 036 private String myStatusMessage; 037 038 public Date getStatusTime() { 039 return myStatusTime; 040 } 041 042 public JobInfo setStatusTime(Date theStatusTime) { 043 myStatusTime = theStatusTime; 044 return this; 045 } 046 047 public BulkImportJobStatusEnum getStatus() { 048 return myStatus; 049 } 050 051 public JobInfo setStatus(BulkImportJobStatusEnum theStatus) { 052 myStatus = theStatus; 053 return this; 054 } 055 056 public String getStatusMessage() { 057 return myStatusMessage; 058 } 059 060 public JobInfo setStatusMessage(String theStatusMessage) { 061 myStatusMessage = theStatusMessage; 062 return this; 063 } 064 } 065 066 /** 067 * Create a new job in {@link ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum#STAGING STAGING} state (meaning it won't yet be worked on and can be added to) 068 */ 069 String createNewJob(BulkImportJobJson theJobDescription, @Nonnull List<BulkImportJobFileJson> theInitialFiles); 070 071 /** 072 * Add more files to a job in {@link ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum#STAGING STAGING} state 073 * 074 * @param theJobId The job ID 075 * @param theFiles The files to add to the job 076 */ 077 void addFilesToJob(String theJobId, List<BulkImportJobFileJson> theFiles); 078 079 /** 080 * Move a job from {@link ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum#STAGING STAGING} 081 * state to {@link ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum#READY READY} 082 * state, meaning that is is a candidate to be picked up for processing 083 * 084 * @param theJobId The job ID 085 */ 086 void markJobAsReadyForActivation(String theJobId); 087 088 /** 089 * This method is intended to be called from the job scheduler, and will begin execution on 090 * the next job in status {@link ca.uhn.fhir.jpa.bulk.imprt.model.BulkImportJobStatusEnum#READY READY} 091 * 092 * @return Returns {@literal true} if a job was activated 093 */ 094 ActivateJobResult activateNextReadyJob(); 095 096 /** 097 * Updates the job status for the given job 098 */ 099 void setJobToStatus(String theJobId, BulkImportJobStatusEnum theStatus); 100 101 /** 102 * Updates the job status for the given job 103 */ 104 void setJobToStatus(String theJobId, BulkImportJobStatusEnum theStatus, String theStatusMessage); 105 106 /** 107 * Gets the job status for the given job. 108 */ 109 JobInfo getJobStatus(String theJobId); 110 111 /** 112 * Gets the number of files available for a given Job ID 113 * 114 * @param theJobId The job ID 115 * @return The file count 116 */ 117 BulkImportJobJson fetchJob(String theJobId); 118 119 /** 120 * Fetch a given file by job ID 121 * 122 * @param theJobId The job ID 123 * @param theFileIndex The index of the file within the job 124 * @return The file 125 */ 126 BulkImportJobFileJson fetchFile(String theJobId, int theFileIndex); 127 128 /** 129 * Delete all input files associated with a particular job 130 */ 131 void deleteJobFiles(String theJobId); 132 133 /** 134 * Fetch just the file description for the given file 135 */ 136 String getFileDescription(String theJobId, int theFileIndex); 137}