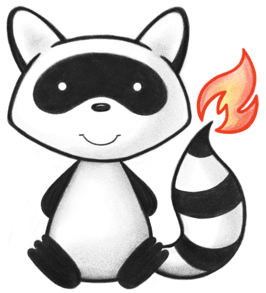
001/*- 002 * #%L 003 * HAPI FHIR Storage api 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.dao.r4; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.jpa.dao.ITransactionProcessorVersionAdapter; 025import ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException; 026import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 027import org.hl7.fhir.exceptions.FHIRException; 028import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 029import org.hl7.fhir.instance.model.api.IBaseResource; 030import org.hl7.fhir.r4.model.Bundle; 031import org.hl7.fhir.r4.model.OperationOutcome; 032import org.hl7.fhir.r4.model.Resource; 033 034import java.util.Date; 035import java.util.List; 036 037public class TransactionProcessorVersionAdapterR4 038 implements ITransactionProcessorVersionAdapter<Bundle, Bundle.BundleEntryComponent> { 039 @Override 040 public void setResponseStatus(Bundle.BundleEntryComponent theBundleEntry, String theStatus) { 041 theBundleEntry.getResponse().setStatus(theStatus); 042 } 043 044 @Override 045 public void setResponseLastModified(Bundle.BundleEntryComponent theBundleEntry, Date theLastModified) { 046 theBundleEntry.getResponse().setLastModified(theLastModified); 047 } 048 049 @Override 050 public void setResource(Bundle.BundleEntryComponent theBundleEntry, IBaseResource theResource) { 051 theBundleEntry.setResource((Resource) theResource); 052 } 053 054 @Override 055 public IBaseResource getResource(Bundle.BundleEntryComponent theBundleEntry) { 056 return theBundleEntry.getResource(); 057 } 058 059 @Override 060 public String getBundleType(Bundle theRequest) { 061 if (theRequest.getType() == null) { 062 return null; 063 } 064 return theRequest.getTypeElement().getValue().toCode(); 065 } 066 067 @Override 068 public void populateEntryWithOperationOutcome( 069 BaseServerResponseException theCaughtEx, Bundle.BundleEntryComponent theEntry) { 070 OperationOutcome oo = new OperationOutcome(); 071 oo.addIssue() 072 .setSeverity(OperationOutcome.IssueSeverity.ERROR) 073 .setDiagnostics(theCaughtEx.getMessage()) 074 .setCode(OperationOutcome.IssueType.EXCEPTION); 075 theEntry.getResponse().setOutcome(oo); 076 } 077 078 @Override 079 public Bundle createBundle(String theBundleType) { 080 Bundle resp = new Bundle(); 081 try { 082 resp.setType(Bundle.BundleType.fromCode(theBundleType)); 083 } catch (FHIRException theE) { 084 throw new InternalErrorException(Msg.code(552) + "Unknown bundle type: " + theBundleType); 085 } 086 return resp; 087 } 088 089 @Override 090 public List<Bundle.BundleEntryComponent> getEntries(Bundle theRequest) { 091 return theRequest.getEntry(); 092 } 093 094 @Override 095 public void addEntry(Bundle theBundle, Bundle.BundleEntryComponent theEntry) { 096 theBundle.addEntry(theEntry); 097 } 098 099 @Override 100 public Bundle.BundleEntryComponent addEntry(Bundle theBundle) { 101 return theBundle.addEntry(); 102 } 103 104 @Override 105 public String getEntryRequestVerb(FhirContext theContext, Bundle.BundleEntryComponent theEntry) { 106 String retVal = null; 107 Bundle.HTTPVerb value = theEntry.getRequest().getMethodElement().getValue(); 108 if (value != null) { 109 retVal = value.toCode(); 110 } 111 return retVal; 112 } 113 114 @Override 115 public String getFullUrl(Bundle.BundleEntryComponent theEntry) { 116 return theEntry.getFullUrl(); 117 } 118 119 @Override 120 public void setFullUrl(Bundle.BundleEntryComponent theEntry, String theFullUrl) { 121 theEntry.setFullUrl(theFullUrl); 122 } 123 124 @Override 125 public String getEntryIfNoneExist(Bundle.BundleEntryComponent theEntry) { 126 return theEntry.getRequest().getIfNoneExist(); 127 } 128 129 @Override 130 public String getEntryRequestUrl(Bundle.BundleEntryComponent theEntry) { 131 return theEntry.getRequest().getUrl(); 132 } 133 134 @Override 135 public void setResponseLocation(Bundle.BundleEntryComponent theEntry, String theResponseLocation) { 136 theEntry.getResponse().setLocation(theResponseLocation); 137 } 138 139 @Override 140 public void setResponseETag(Bundle.BundleEntryComponent theEntry, String theEtag) { 141 theEntry.getResponse().setEtag(theEtag); 142 } 143 144 @Override 145 public String getEntryRequestIfMatch(Bundle.BundleEntryComponent theEntry) { 146 return theEntry.getRequest().getIfMatch(); 147 } 148 149 @Override 150 public String getEntryRequestIfNoneExist(Bundle.BundleEntryComponent theEntry) { 151 return theEntry.getRequest().getIfNoneExist(); 152 } 153 154 @Override 155 public String getEntryRequestIfNoneMatch(Bundle.BundleEntryComponent theEntry) { 156 return theEntry.getRequest().getIfNoneMatch(); 157 } 158 159 @Override 160 public void setResponseOutcome(Bundle.BundleEntryComponent theEntry, IBaseOperationOutcome theOperationOutcome) { 161 theEntry.getResponse().setOutcome((Resource) theOperationOutcome); 162 } 163 164 @Override 165 public void setRequestVerb(Bundle.BundleEntryComponent theEntry, String theVerb) { 166 theEntry.getRequest().setMethod(Bundle.HTTPVerb.fromCode(theVerb)); 167 } 168 169 @Override 170 public void setRequestUrl(Bundle.BundleEntryComponent theEntry, String theUrl) { 171 theEntry.getRequest().setUrl(theUrl); 172 } 173}